狂神JUC并发
开始之前:进程和线程的区别?
进程是操作系统进行资源分配和调度的基本单位,是一个程序的运行实例,拥有独立的内存空间。
一个进程可以包含多个线程,是CPU调度和执行的最小单位,可以共享属于同一进程的资源。简而言之,进程间相互独立,而线程间可以共享内存和资源。
准备工作
新建一个Maven项目,引入一个lombok依赖.
<dependencies>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.8</version>
</dependency>
</dependencies>
1. 什么是JUC
JUC就是java.util.concurrent下面的类包,专门用于多线程的开发。
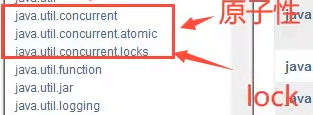
1.1 进程
一个程序,QQ.exe Music.exe 程序的集合;
一个进程往往可以包含多个线程,至少包含一个!
Java默认有几个线程? 2 个 mian、GC
1.2 线程
开了一个进程 Typora,写字,自动保存(线程负责的)
对于Java而言:Thread、Runable、Callable进行开启线程的。
提问?JAVA真的可以开启线程吗? 开不了的!
Java是没有权限去开启线程、操作硬件的,这是一个native的一个本地方法,它调用的底层的C++代码。
public synchronized void start() {
/**
* This method is not invoked for the main method thread or "system"
* group threads created/set up by the VM. Any new functionality added
* to this method in the future may have to also be added to the VM.
*
* A zero status value corresponds to state "NEW".
*/
if (threadStatus != 0)
throw new IllegalThreadStateException();
/* Notify the group that this thread is about to be started
* so that it can be added to the group's list of threads
* and the group's unstarted count can be decremented. */
group.add(this);
boolean started = false;
try {
start0();
started = true;
} finally {
try {
if (!started) {
group.threadStartFailed(this);
}
} catch (Throwable ignore) {
/* do nothing. If start0 threw a Throwable then
it will be passed up the call stack */
}
}
}
//这是一个C++底层,Java是没有权限操作底层硬件的
private native void start0();
首先。start()是一个synchronized方法,同步方法,安全的方法,这个方法会把当前线程加入一个线程组,调用了start0()方法,这个start0(),是用native修饰的,也就是本地方法。
所以最后是调用了本地方法,JAVA是没有权限开启线程的。start()调用了本地的C++方法,因为java是运行在虚拟机之上的,无法直接操作硬件
1.3 并发
多线程操作同一个资源。
- CPU 只有一核,模拟出来多条线程,天下武功,唯快不破。那么我们就可以使用CPU快速交替,来模拟多线程。
- 并发编程的本质:充分利用CPU的资源!
1.4 并行
并行: 多个人一起行走
- CPU多核,多个线程可以同时执行。 我们可以使用线程池!
获取cpu的核数
public class Test1 {
public static void main(String[] args) {
//获取cpu的核数
System.out.println(Runtime.getRuntime().availableProcessors());
}
}
1.5 线程的状态
public enum State {
//新生
NEW,
//运行
RUNNABLE,
//阻塞
BLOCKED,
//等待
WAITING,
//超时等待
TIMED_WAITING,
//终止
TERMINATED;
}
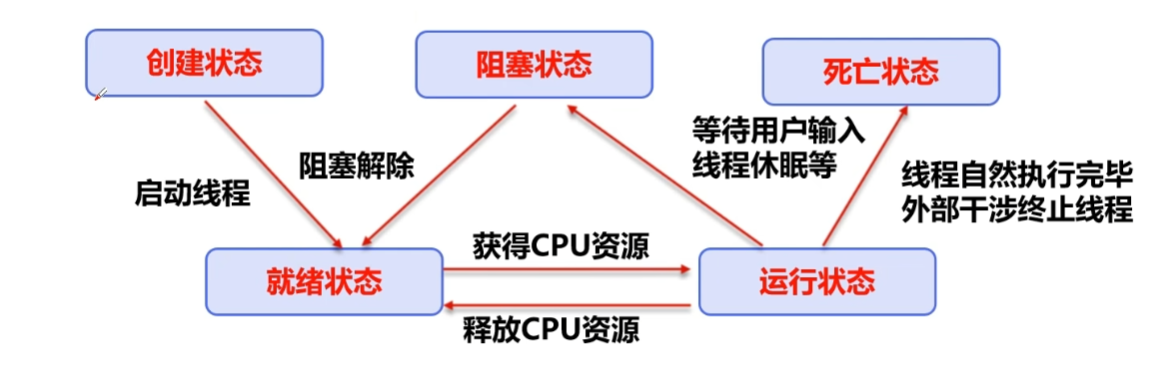
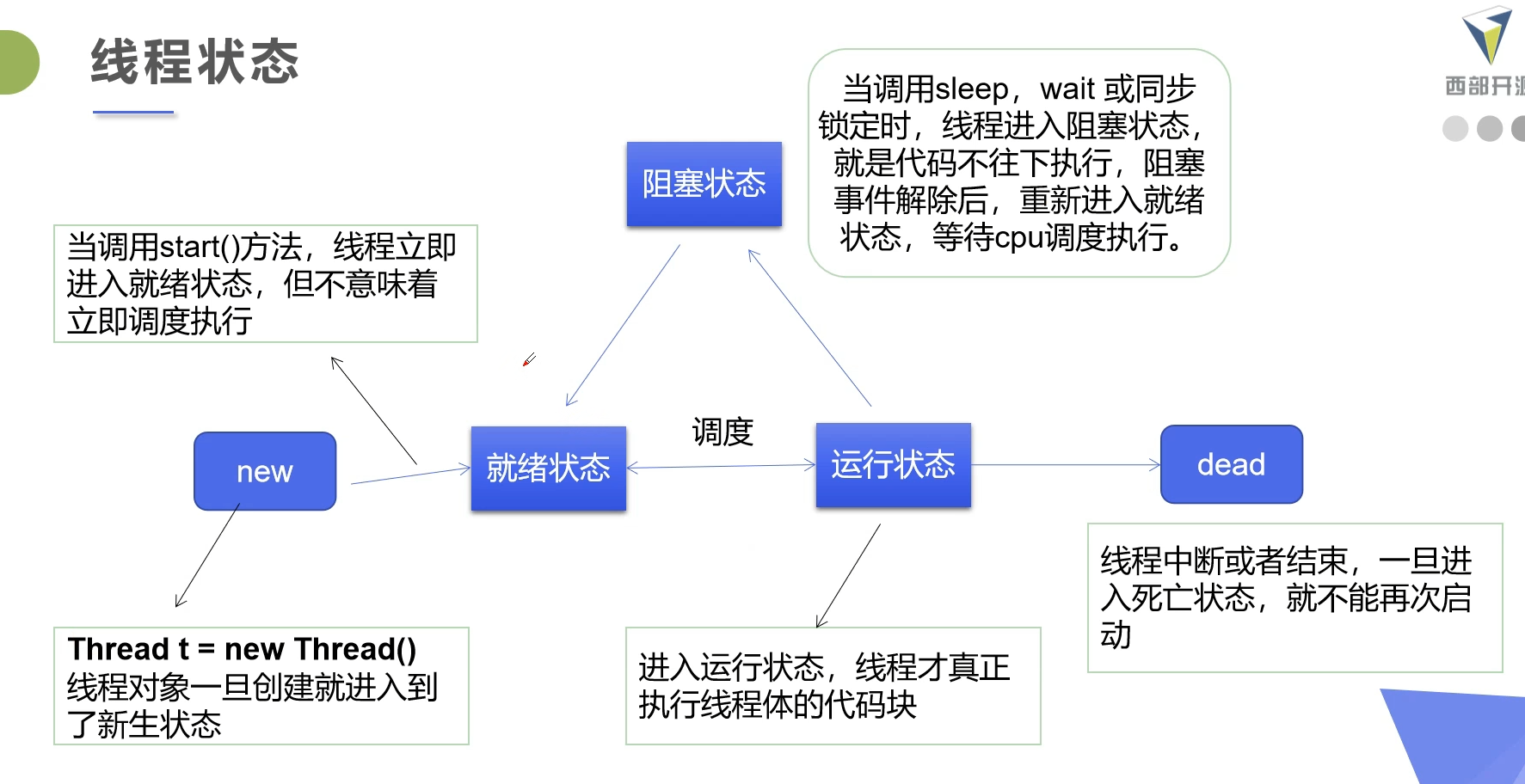
1.7 线程run/start的区别
run是线程普通方法,线程实际执行运行的代码,start是启动方法,启动方法中会调用run方法
1.7 wait/sleep的区别
1、来自不同的类
wait => Object
sleep => Thread
一般情况企业中使用休眠是:
TimeUnit.DAYS.sleep(1); //休眠1天
TimeUnit.SECONDS.sleep(1); //休眠1s
2、关于锁的释放
wait 会释放对象的锁,使得当前线程进入等待状态,直到其他线程调用notify()或notifyAll()方法唤醒;
sleep使得当前线程暂停执行一段时间,不会释放锁;
3、使用的范围是不同的
wait 必须在synchronized同步代码块中;
sleep 可以在任何地方睡!
4、是否需要捕获异常
wait是不需要捕获异常;
sleep必须要捕获异常;
5、使用场景不同
wait用于多个线程之间的协作通信,sleep用于线程的休眠
2.Lock
2.1 传统的 synchronized
//基本的卖票例子
//真正的多线程开发,公司中的开发,降低耦合性
//线程就是一个单独的资源类,没有任何附属的操作!
//1、属性、方法
public class SaleTicketDemo01 {
public static void main(String[] args) {
//并发:多线程操作同一个资源类,把资源类丢入线程
final Ticket ticket = new Ticket();
///@FunctionalInterface函数式接口,jdk1.8 Lambda表达式(参数)->{ 代码 }
new Thread(()->{
for (int i = 0; i < 40; i++) {
ticket.sale();
}
},"A").start();
new Thread(()->{
for (int i = 0; i < 40; i++) {
ticket.sale();
}
},"B").start();
new Thread(()->{
for (int i = 0; i < 40; i++) {
ticket.sale();
}
},"C").start();
}
}
// 资源类 OOP 属性、方法
class Ticket {
private int number = 30;
//卖票的方式
public synchronized void sale() {
if (number > 0) {
System.out.println(Thread.currentThread().getName() + "卖出了第" + (number--) + "张票剩余" + number + "张票");
}
}
}
2.2 Lock

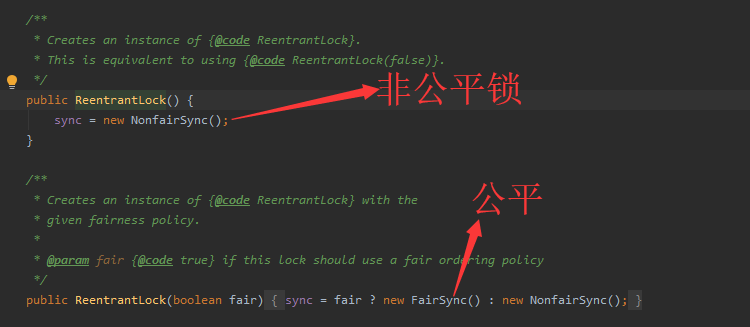
公平锁:十分公平,先来后到,排队.
非公平锁:不公平,可以插队
默认是非公平锁,是为了公平,比如一个线程要3s,另一个线程要3h,难道一定要让3h的锁先来就先执行吗
public class SaleTicketDemo02 {
public static void main(String[] args) {
final Ticket ticket = new Ticket();
new Thread(()->{
for (int i = 0; i < 40; i++) {
ticket.sale();
}
},"A").start();
new Thread(()->{
for (int i = 0; i < 40; i++) {
ticket.sale();
}
},"B").start();
new Thread(()->{
for (int i = 0; i < 40; i++) {
ticket.sale();
}
},"C").start();
}
}
// 资源类 OOP 属性、方法
//Lock三部曲
//1、new ReentrantLock();
//2、Lock.Lock();//加锁
//3、finally=>Lock.unLock(); //解锁
class Ticket2 {
private int number = 30;
Lock lock=new ReentrantLock();
//卖票的方式
public void sale() {
lock.lock();
try {
if (number > 0) {
System.out.println(Thread.currentThread().getName() + "卖出了第" + (number--) + "张票剩余" + number + "张票");
}
} catch (Exception e) {
e.printStackTrace();
} finally {
lock.unlock();
}
}
}
2.3 Synchronized 与Lock 的区别
1、Synchronized 内置的Java关键字,Lock是一个Java接口,有很多实现
2、Synchronized 无法判断获取锁的状态,Lock可以判断(提供了 tryLock()
方法,可以尝试获取锁并返回成功或失败)
3、Synchronized 会自动释放锁,lock必须要手动加锁和手动释放锁!可能会遇到死锁
4、Synchronized
在获取锁时,如果锁被占用,线程会一直阻塞等待。;lock就不一定会一直等待下去,lock会有一个trylock去尝试获取锁,若失败不会一直等待,提供了更大的灵活性。
5、synchronized
是非公平锁,等待锁的线程不保证按照先来后到的顺序获取锁。lock
可以通过构造函数设置为公平锁(new ReentrantLock(true)
),保证等待时间最长的线程优先获取锁。
6、Synchronized 适合锁少量的代码同步问题,Lock适合锁大量的同步代码;
2.4 生产者和消费者的关系
面试高频: 单例模式,生产者消费者,死锁
1)Synchronzied 版本
/*
线程之间的通信问题:生产者和消费者问题
线程交替执行 A B操作同一个变量 num=0 A让num+1,B让num-1;
**/
public class ConsumeAndProduct {
public static void main(String[] args) {
Data data = new Data();
new Thread(() -> {
for (int i = 0; i < 10; i++) {
try {
data.increment();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}, "A").start();
new Thread(() -> {
for (int i = 0; i < 10; i++) {
try {
data.decrement();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}, "B").start();
}
}
// 等待,业务,通知
class Data {
private int num = 0;
// +1
public synchronized void increment() throws InterruptedException {
// 判断需不需要等待
if (num != 0) { //不等于0,等待被人减掉; 如果为0,那就加上;
this.wait();
}
num++;
System.out.println(Thread.currentThread().getName() + "=>" + num);
// 通知其他线程 +1 执行完毕
this.notifyAll();
}
// -1
public synchronized void decrement() throws InterruptedException {
// 判断等待
if (num == 0) {
this.wait();
}
num--;
System.out.println(Thread.currentThread().getName() + "=>" + num);
// 通知其他线程 -1 执行完毕
this.notifyAll();
}
}
2)存在问题(虚假唤醒)
问题,如果有四个线程,会出现虚假唤醒
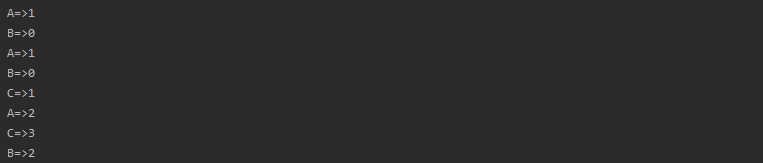
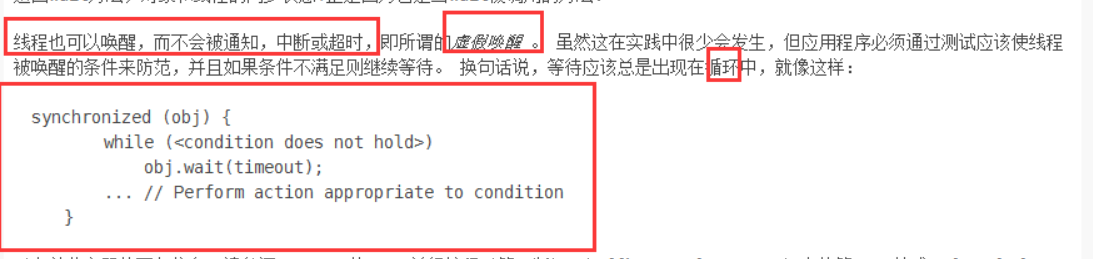
解决方式 ,if 改为while即可,防止虚假唤醒
结论:就是用if判断的话,唤醒后线程会从wait之后的代码开始运行,但是不会重新判断if条件,直接继续运行if代码块之后的代码,而如果使用while的话,也会从wait之后的代码运行,但是唤醒后会重新判断循环条件,如果不成立再执行while代码块之后的代码块,成立的话继续wait。
这也就是为什么用while而不用if的原因了,因为线程被唤醒后,执行开始的地方是wait之后
if情况:被唤醒时,无脑执行后面代码
while:还会再次判断一下
3)Lock版
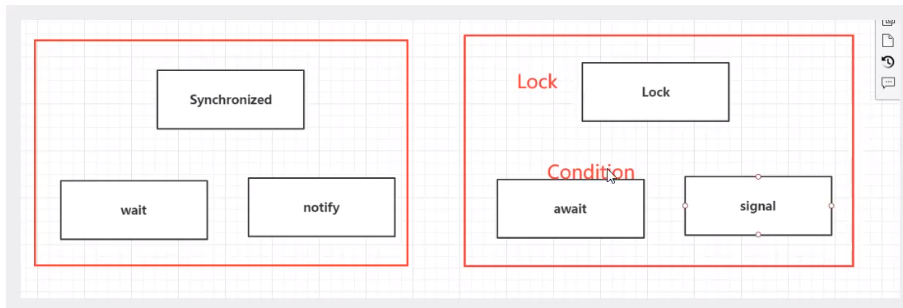
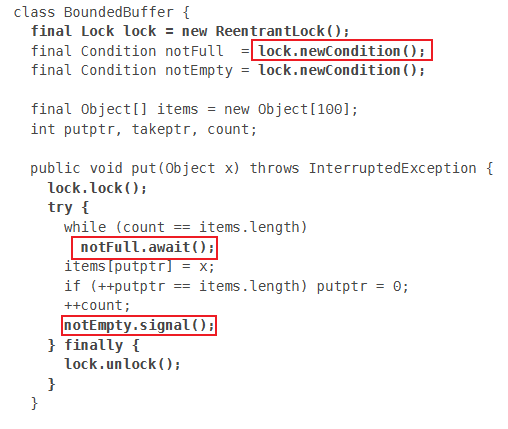
public class LockCAP {
public static void main(String[] args) {
Data2 data = new Data2();
new Thread(() -> {
for (int i = 0; i < 10; i++) {
try {
data.increment();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}, "A").start();
new Thread(() -> {
for (int i = 0; i < 10; i++) {
try {
data.decrement();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}, "B").start();
new Thread(() -> {
for (int i = 0; i < 10; i++) {
try {
data.increment();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}, "C").start();
new Thread(() -> {
for (int i = 0; i < 10; i++) {
try {
data.decrement();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}, "D").start();
}
}
class Data2 {
private int num = 0;
Lock lock = new ReentrantLock();
Condition condition = lock.newCondition();
// +1
public void increment() throws InterruptedException {
lock.lock();
try {
// 判断等待
while (num != 0) {
condition.await(); //等待
}
num++;
System.out.println(Thread.currentThread().getName() + "=>" + num);
// 通知其他线程 +1 执行完毕
condition.signalAll(); //唤醒全部
}finally {
lock.unlock();
}
}
// -1
public void decrement() throws InterruptedException {
lock.lock();
try {
// 判断等待
while (num == 0) {
condition.await();
}
num--;
System.out.println(Thread.currentThread().getName() + "=>" + num);
// 通知其他线程 +1 执行完毕
condition.signalAll();
}finally {
lock.unlock();
}
}
}
4)Condition的优势
精准的通知和唤醒的线程!
如果我们要指定通知的下一个进行顺序怎么办呢? 我们可以使用Condition来指定通知进程~
任何一个新的技术,绝对不是仅仅只是覆盖了原来的技术,优势和补充!
/**
* Description:
* A 执行完 调用B
* B 执行完 调用C
* C 执行完 调用A
*
* @author jiaoqianjin
* Date: 2020/8/11 9:58
**/
public class C {
public static void main(String[] args) {
Data3 data3 = new Data3();
new Thread(() -> {
for (int i = 0; i < 10; i++) {
data3.printA();
}
},"A").start();
new Thread(() -> {
for (int i = 0; i < 10; i++) {
data3.printB();
}
},"B").start();
new Thread(() -> {
for (int i = 0; i < 10; i++) {
data3.printC();
}
},"C").start();
}
}
class Data3 {
private Lock lock = new ReentrantLock();
private Condition condition1 = lock.newCondition();
private Condition condition2 = lock.newCondition();
private Condition condition3 = lock.newCondition();
private int num = 1; // 1A 2B 3C
public void printA() {
lock.lock();
try {
// 业务代码 判断 -> 执行 -> 通知
while (num != 1) {
condition1.await();
}
System.out.println(Thread.currentThread().getName() + "==> AAAA" );
num = 2;
condition2.signal();
}catch (Exception e) {
e.printStackTrace();
}finally {
lock.unlock();
}
}
public void printB() {
lock.lock();
try {
// 业务代码 判断 -> 执行 -> 通知
while (num != 2) {
condition2.await();
}
System.out.println(Thread.currentThread().getName() + "==> BBBB" );
num = 3;
condition3.signal();
}catch (Exception e) {
e.printStackTrace();
}finally {
lock.unlock();
}
}
public void printC() {
lock.lock();
try {
// 业务代码 判断 -> 执行 -> 通知
while (num != 3) {
condition3.await();
}
System.out.println(Thread.currentThread().getName() + "==> CCCC" );
num = 1;
condition1.signal();
}catch (Exception e) {
e.printStackTrace();
}finally {
lock.unlock();
}
}
}
/*
A==> AAAA
B==> BBBB
C==> CCCC
A==> AAAA
B==> BBBB
C==> CCCC
...
*/
2.5 8锁现象
如何判断锁的是谁!锁到底锁的是谁?
锁会锁住:对象、Class
深刻理解我们的锁
问题1
两个同步方法,先执行发短信还是打电话
public class dome01 {
public static void main(String[] args) {
Phone phone = new Phone();
new Thread(() -> { phone.sendMs(); }).start();
TimeUnit.SECONDS.sleep(1);
new Thread(() -> { phone.call(); }).start();
}
}
class Phone {
public synchronized void sendMs() {
System.out.println("发短信");
}
public synchronized void call() {
System.out.println("打电话");
}
}
输出结果为
发短信
打电话
为什么? 如果你认为每次都不一样? 这个答案是错误的!
问题2:
我们再来看:我们让发短信 延迟4s
public class dome0 {
public static void main(String[] args) throws InterruptedException {
Phone phone = new Phone();
new Thread(() -> {
try {
phone.sendMs();
} catch (InterruptedException e) {
e.printStackTrace();
}
}).start();
TimeUnit.SECONDS.sleep(1);
new Thread(() -> { phone.call(); }).start();
}
}
class Phone {
public synchronized void sendMs() throws InterruptedException {
TimeUnit.SECONDS.sleep(4);
System.out.println("发短信");
}
public synchronized void call() {
System.out.println("打电话");
}
}
现在结果是什么呢?
结果:还是先发短信,然后再打电话!
原因:并不是顺序执行,而是synchronized 锁住的对象是方法的调用者,也就是
phone
!对于两个方法用的是同一个锁,谁先拿到谁先执行,另外一个等待
问题三
加一个普通方法
public class dome03 {
public static void main(String[] args) throws InterruptedException {
Phone phone = new Phone();
new Thread(() -> {
try {
phone.sendMs();
} catch (InterruptedException e) {
e.printStackTrace();
}
}).start();
TimeUnit.SECONDS.sleep(1);
new Thread(() -> { phone.hello(); }).start();
}
}
class Phone {
public synchronized void sendMs() throws InterruptedException {
TimeUnit.SECONDS.sleep(4);
System.out.println("发短信");
}
public synchronized void call() {
System.out.println("打电话");
}
public void hello() {
System.out.println("hello");
}
}
输出结果为
hello
发短信
原因:hello是一个普通方法,不受synchronized锁的影响,不用等待锁的释放
问题四
如果我们使用的是两个对象,一个调用发短信,一个调用打电话,那么整个顺序是怎么样的呢?
public class dome04 {
public static void main(String[] args) throws InterruptedException {
Phone phone1 = new Phone();
Phone phone2 = new Phone();
new Thread(() -> {
try {
phone1.sendMs();
} catch (InterruptedException e) {
e.printStackTrace();
}
}).start();
TimeUnit.SECONDS.sleep(1);
new Thread(() -> { phone2.call(); }).start();
}
}
class Phone {
public synchronized void sendMs() throws InterruptedException {
TimeUnit.SECONDS.sleep(4);
System.out.println("发短信");
}
public synchronized void call() {
System.out.println("打电话");
}
public void hello() {
System.out.println("hello");
}
}
输出结果
打电话
发短信
原因:两个对象两把锁,不会出现等待的情况,发短信睡了4s,所以先执行打电话
问题五、六
如果我们把synchronized的方法加上static变成静态方法!那么顺序又是怎么样的呢?
(1)我们先来使用一个对象调用两个方法!
答案是:先发短信,后打电话
(2)如果我们使用两个对象调用两个方法!
答案是:还是先发短信,后打电话
原因是什么呢? 为什么加了static就始终前面一个对象先执行呢!为什么后面会等待呢?
原因是:对于static静态方法来说,对于整个类Class来说只有一份,类一加载就有了,对于不同的对象使用的是同一份方法,相当于这个方法是属于这个类的,如果静态static方法使用synchronized锁定,那么这个synchronized锁会锁住整个对象!不管多少个对象,对于静态的锁都只有一把锁,谁先拿到这个锁就先执行,其他的进程都需要等待!
问题七
如果我们使用一个静态同步方法、一个同步方法、一个对象调用顺序是什么?
public class dome07 {
public static void main(String[] args) throws InterruptedException {
Phone phone1 = new Phone();
// Phone phone2 = new Phone();
new Thread(() -> {
try {
phone1.sendMs();
} catch (InterruptedException e) {
e.printStackTrace();
}
}).start();
TimeUnit.SECONDS.sleep(1);
new Thread(() -> { phone1.call(); }).start();
}
}
class Phone {
public static synchronized void sendMs() throws InterruptedException {
TimeUnit.SECONDS.sleep(4);
System.out.println("发短信");
}
public synchronized void call() {
System.out.println("打电话");
}
public void hello() {
System.out.println("hello");
}
}
输出结果
打电话
发短信
原因:因为一个锁的是Class类的模板,一个锁的是对象的调用者。所以不存在等待,直接运行。
问题八
如果我们使用一个静态同步方法、一个同步方法、两个对象调用顺序是什么?
public class dome08 {
public static void main(String[] args) throws InterruptedException {
Phone phone1 = new Phone();
Phone phone2 = new Phone();
new Thread(() -> {
try {
phone1.sendMs();
} catch (InterruptedException e) {
e.printStackTrace();
}
}).start();
TimeUnit.SECONDS.sleep(1);
new Thread(() -> { phone2.call(); }).start();
}
}
class Phone {
public static synchronized void sendMs() throws InterruptedException {
TimeUnit.SECONDS.sleep(4);
System.out.println("发短信");
}
public synchronized void call() {
System.out.println("打电话");
}
public void hello() {
System.out.println("hello");
}
}
输出结果
打电话
发短信
原因:两把锁锁的不是同一个东西
小结
new 出来的 this 是具体的一个对象(手机)
static Class 是唯一的一个模板
3. 集合不安全
3.1 List 不安全
//java.util.ConcurrentModificationException 并发修改异常!
public class ListTest {
public static void main(String[] args) {
List<Object> arrayList = new ArrayList<>();
for(int i=1;i<=10;i++){
new Thread(()->{
arrayList.add(UUID.randomUUID().toString().substring(0,5));
System.out.println(arrayList);
},String.valueOf(i)).start();
}
}
}
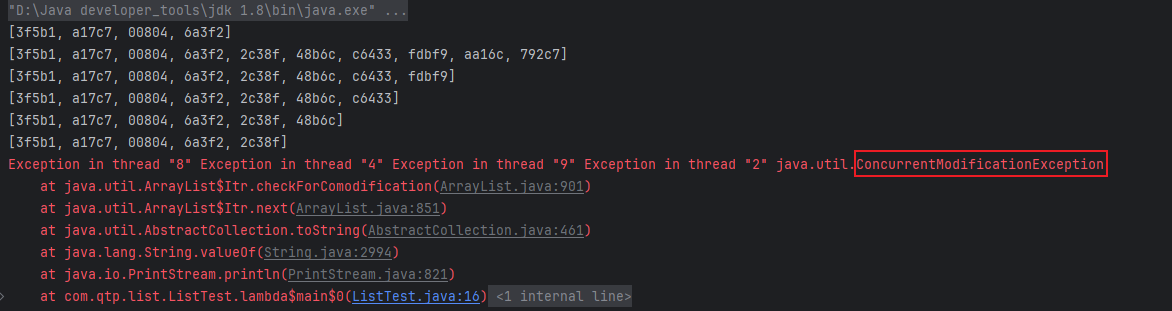
会导致 java.util.ConcurrentModificationException 并发修改异常!
ArrayList 在并发情况下是不安全的
ArrayList
没有同步开销,性能更高,在单线程环境下,ArrayList
是更好的选择;如果需要线程安全,可以使用 Collections.synchronizedList()
或 CopyOnWriteArrayList
。
解决方案:
public class ListTest2 {
public static void main(String[] args) {
/**
* 解决方案
* 1. List<String> list = new Vector<>();
* 2. List<String> list = Collections.synchronizedList(new ArrayList<>());
* 3. List<String> list = new CopyOnWriteArrayList<>();
*/
List<String> list = new CopyOnWriteArrayList<>();
for (int i = 1; i <=10; i++) {
new Thread(() -> {
list.add(UUID.randomUUID().toString().substring(0,5));
System.out.println(list);
},String.valueOf(i)).start();
}
}
}
CopyOnWriteArrayList:写入时复制! COW 计算机程序设计领域的一种优化策略
核心思想是,如果有多个调用者(Callers)同时要求相同的资源(如内存或者是磁盘上的数据存储),他们会共同获取相同的指针指向相同的资源,直到某个调用者视图修改资源内容时,系统才会真正复制一份专用副本(private copy)给该调用者,而其他调用者所见到的最初的资源仍然保持不变。这过程对其他的调用者都是透明的(transparently)。此做法主要的优点是如果调用者没有修改资源,就不会有副本(private copy)被创建,因此多个调用者只是读取操作时可以共享同一份资源。
读的时候不需要加锁,如果读的时候有多个线程正在向CopyOnWriteArrayList添加数据,读还是会读到旧的数据,因为写的时候不会锁住旧的CopyOnWriteArrayList。
多个线程调用的时候,list,读取的时候,固定的,写入(存在覆盖操作);在写入的时候避免覆盖,造成数据错乱的问题;
CopyOnWriteArrayList比Vector厉害在哪里?
Vector底层是使用synchronized关键字来实现的:效率特别低下。
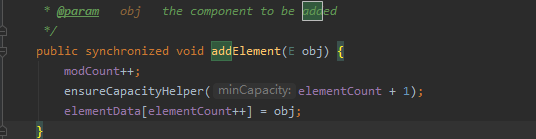
CopyOnWriteArrayList使用的是Lock锁,效率会更加高效!
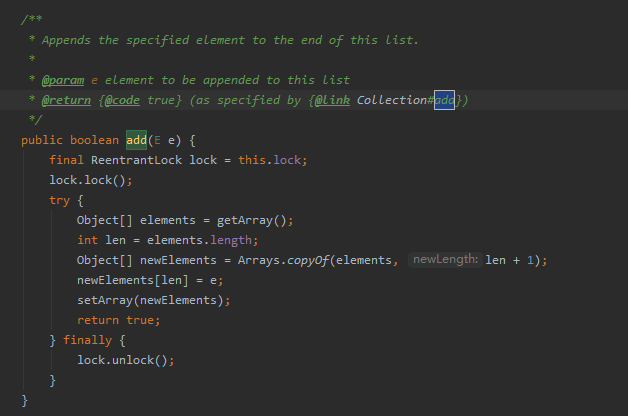
3.1 set 不安全
Set和List同理可得: 多线程情况下,普通的Set集合是线程不安全的;
解决方案还是两种:
- 使用Collections工具类的synchronized包装的Set类
- 使用CopyOnWriteArraySet 写入复制的JUC解决方案
public class SetTest {
public static void main(String[] args) {
/**
* 1. Set<String> set = Collections.synchronizedSet(new HashSet<>());
* 2. Set<String> set = new CopyOnWriteArraySet<>();
*/
// Set<String> set = new HashSet<>();
Set<String> set = new CopyOnWriteArraySet<>();
for (int i = 1; i <= 30; i++) {
new Thread(() -> {
set.add(UUID.randomUUID().toString().substring(0,5));
System.out.println(set);
},String.valueOf(i)).start();
}
}
}
HashSet底层是什么?
hashSet底层就是一个HashMap;
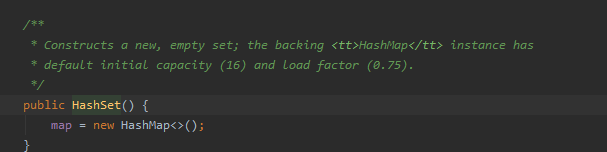
3.3 Map不安全
//map 是这样用的吗? 不是,工作中不使用这个
//默认等价什么? new HashMap<>(16,0.75);
Map<String, String> map = new HashMap<>();
//加载因子、初始化容量
默认加载因子是0.75,默认的初始容量是16
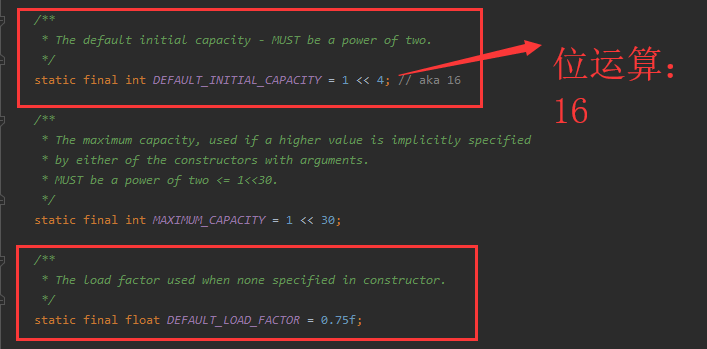
同样的HashMap基础类也存在并发修改异常!
public class MapTest {
public static void main(String[] args) {
//map 是这样用的吗? 不是,工作中不使用这个
//默认等价什么? new HashMap<>(16,0.75);
/**
* 解决方案
* 1. Map<String, String> map = Collections.synchronizedMap(new HashMap<>());
* Map<String, String> map = new ConcurrentHashMap<>();
*/
Map<String, String> map = new ConcurrentHashMap<>();
//加载因子、初始化容量
for (int i = 1; i < 100; i++) {
new Thread(()->{
map.put(Thread.currentThread().getName(), UUID.randomUUID().toString().substring(0,5));
System.out.println(map);
},String.valueOf(i)).start();
}
}
}
4. Callable
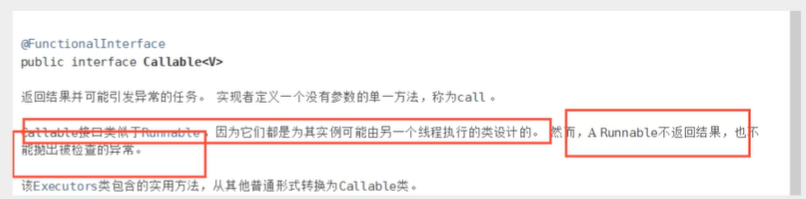
1、可以有返回值;
2、可以抛出异常;
3、方法不同,run()/call()
- 在循环中,每次创建一个
MyThread1
实例,并将其包装到FutureTask
中。 - 将
FutureTask
放入Thread
中启动线程。 - 调用
futureTask.get()
获取call
方法的返回值。
package com.qtp.callable;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.FutureTask;
public class CallableTest {
public static void main(String[] args) throws ExecutionException, InterruptedException {
// for (int i = 1; i < 10; i++) {
// MyThread1 myThread1 = new MyThread1();
//
// FutureTask<Integer> futureTask = new FutureTask<>(myThread1);
// // 放入Thread中使用,结果会被缓存
// new Thread(futureTask,String.valueOf(i)).start();
// // 这个get方法可能会被阻塞,如果在call方法中是一个耗时的方法,所以一般情况我们会把这个放在最后,或者使用异步通信
// int a = futureTask.get();
// System.out.println("返回值:" + a);
// }
MyThread1 myThread1 = new MyThread1();
FutureTask<String> futureTask = new FutureTask<>(myThread1);
new Thread(futureTask,"A").start();
new Thread(futureTask,"B").start();
System.out.println(futureTask.get());//这个get方法可能会产生阻塞!把他放到最后,或者使用异步通信来处理
}
}
class MyThread1 implements Callable<String> {
@Override
public String call() throws Exception {
System.out.println("当前线程名称: " + Thread.currentThread().getName());
return 1024+":"+Thread.currentThread().getName();
}
}

如果多个线程共享同一个 FutureTask
实例,FutureTask
内部的 call
方法只会执行一次。这是因为 FutureTask
的设计是为了确保任务只执行一次,并将结果缓存起来,供后续的 get
方法调用。
细节:
- 有缓存
- 结果可能需要等待,会阻塞
5. 常用的辅助类
1)CountDownLatch
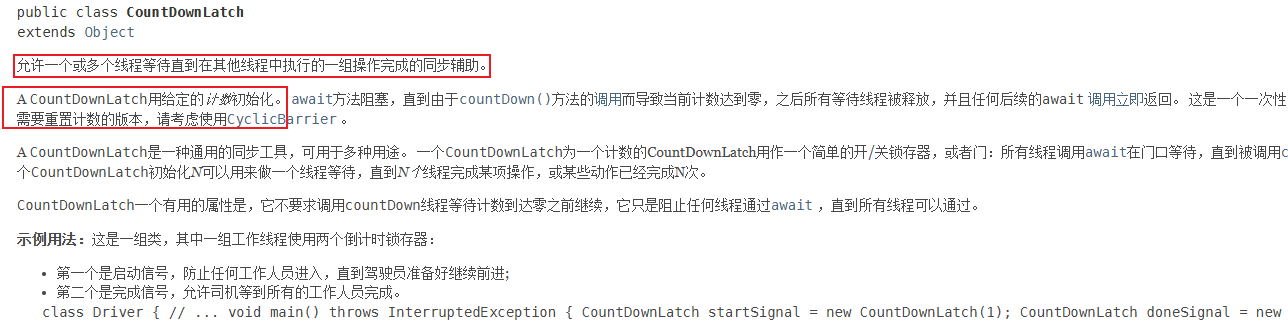
public class CountDownLatchDemo {
public static void main(String[] args) throws InterruptedException {
// 总数是6
CountDownLatch countDownLatch = new CountDownLatch(6);
for (int i = 1; i <= 6; i++) {
new Thread(() -> {
System.out.println(Thread.currentThread().getName() + "==> Go Out");
countDownLatch.countDown(); // 每个线程都数量 -1
},String.valueOf(i)).start();
}
countDownLatch.await(); // 等待计数器归零 然后向下执行
System.out.println("close door");
}
}
主要方法:
- countDown 减一操作;
- await 等待计数器归零
await 等待计数器归零,就唤醒,再继续向下运行
如果计数器是6,只减了5次,那会一直等待。
2)CyclickBarrier

public class CyclicBarrierDemo {
public static void main(String[] args) {
// 主线程
CyclicBarrier cyclicBarrier = new CyclicBarrier(7,() -> {
System.out.println("召唤神龙");
});
for (int i = 1; i <= 7; i++) {
// 子线程
int finalI = i;
new Thread(() -> {
System.out.println(Thread.currentThread().getName() + "收集了第" + finalI + "颗龙珠");
try {
/**
每个线程在执行到 await() 方法时,会阻塞并等待其他线程也调用 await() 方法。每当一个线程调 用 await() 方法时,CyclicBarrier 的内部计数器会减一(而不是加一),直到计数器减到 0,屏 障才会打开。
*/
cyclicBarrier.await(); // 加法计数 等待
} catch (InterruptedException e) {
e.printStackTrace();
} catch (BrokenBarrierException e) {
e.printStackTrace();
}
}).start();
}
}
}
3)Semaphore
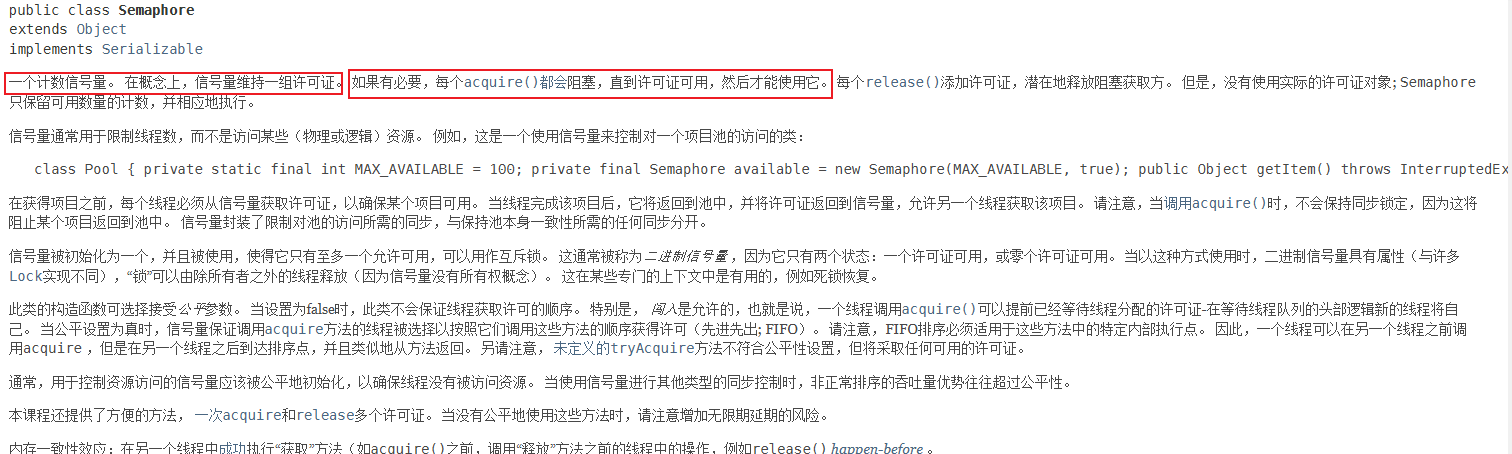
public class SemaphoreDemo {
public static void main(String[] args) {
// 线程数量,停车位,限流
// 有限的条件下,有序!
Semaphore semaphore = new Semaphore(3);
for (int i = 0; i <= 6; i++) {
new Thread(() -> {
// acquire() 得到
try {
semaphore.acquire();
System.out.println(Thread.currentThread().getName() + "抢到车位");
TimeUnit.SECONDS.sleep(2);
System.out.println(Thread.currentThread().getName() + "离开车位");
}catch (Exception e) {
e.printStackTrace();
}finally {
semaphore.release(); // release() 释放
}
}).start();
}
}
}
原理:
semaphore.acquire()获得资源,如果资源已经使用完了,就等待资源释放后再进行使用!
semaphore.release()释放,会将当前的信号量释放+1,然后唤醒等待的线程!
作用: 多个共享资源互斥的使用! 并发限流,控制最大的线程数!
6. 读写锁
public class ReadWriteLockDemo {
public static void main(String[] args) {
MyCache myCache = new MyCache();
int num = 6;
for (int i = 1; i <= num; i++) {
int finalI = i;
new Thread(() -> {
myCache.write(String.valueOf(finalI), String.valueOf(finalI));
},String.valueOf(i)).start();
}
for (int i = 1; i <= num; i++) {
int finalI = i;
new Thread(() -> {
myCache.read(String.valueOf(finalI));
},String.valueOf(i)).start();
}
}
}
/**
* 方法未加锁,导致写的时候被插队
*/
class MyCache {
private volatile Map<String, String> map = new HashMap<>();
public void write(String key, String value) {
System.out.println(Thread.currentThread().getName() + "线程开始写入");
map.put(key, value);
System.out.println(Thread.currentThread().getName() + "线程写入ok");
}
public void read(String key) {
System.out.println(Thread.currentThread().getName() + "线程开始读取");
map.get(key);
System.out.println(Thread.currentThread().getName() + "线程写读取ok");
}
}
2线程开始写入
2线程写入ok
3线程开始写入
3线程写入ok
1线程开始写入 # 插入了其他线程的写入,导致数据不一致
4线程开始写入
4线程写入ok
1线程写入ok
6线程开始写入
6线程写入ok
5线程开始写入
5线程写入ok
1线程开始读取
1线程写读取ok
2线程开始读取
2线程写读取ok
3线程开始读取
3线程写读取ok
4线程开始读取
4线程写读取ok
5线程开始读取
6线程开始读取
6线程写读取ok
5线程写读取ok
Process finished with exit code 0
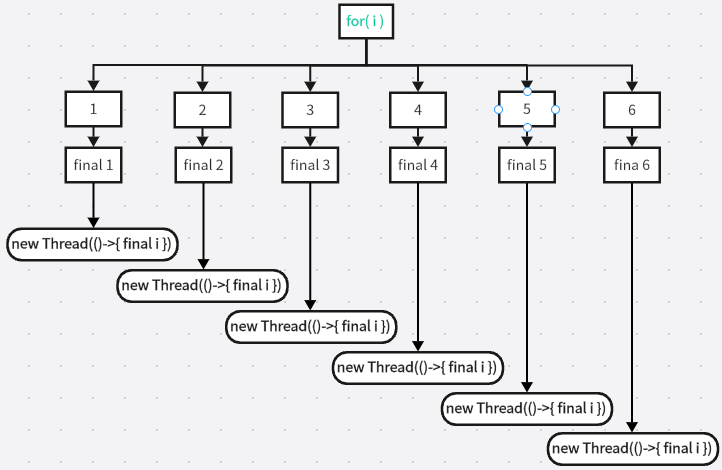
所以如果我们不加锁的情况,多线程的读写会造成数据不可靠的问题。
你写入1的时候,写如ok也要输出才算完成,但是这个时候插入进来了一个线程2。
我们也可以采用synchronized这种重量锁和轻量锁 lock去保证数据的可靠。
但是这次我们采用更细粒度的锁:ReadWriteLock 读写锁来保证
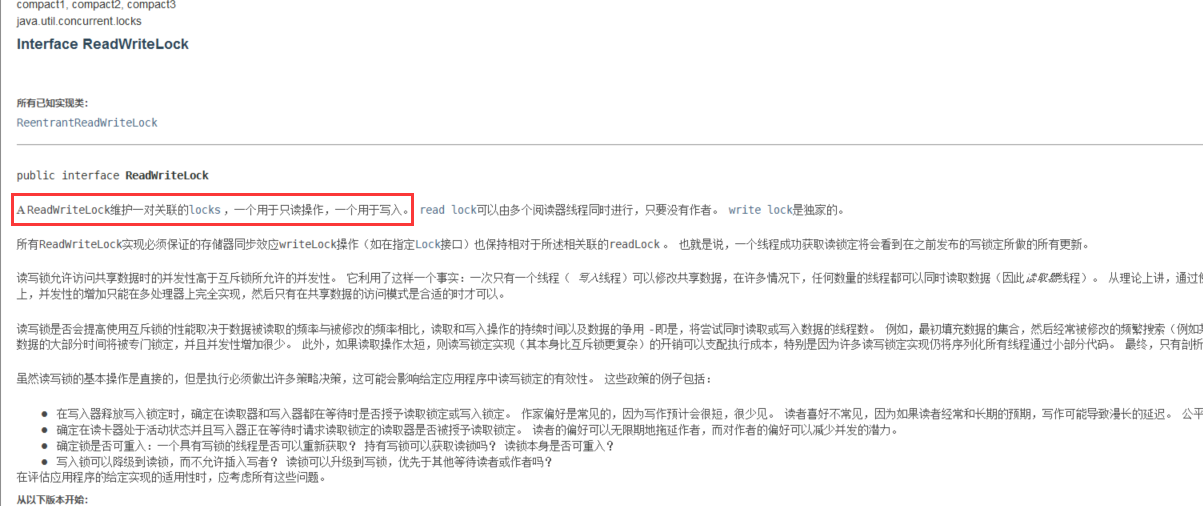
public class ReadWriteLockDemo {
public static void main(String[] args) {
MyCache2 myCache = new MyCache2();
int num = 6;
for (int i = 1; i <= num; i++) {
int finalI = i;
new Thread(() -> {
myCache.write(String.valueOf(finalI), String.valueOf(finalI));
},String.valueOf(i)).start();
}
for (int i = 1; i <= num; i++) {
int finalI = i;
new Thread(() -> {
myCache.read(String.valueOf(finalI));
},String.valueOf(i)).start();
}
}
}
class MyCache2 {
private volatile Map<String, String> map = new HashMap<>();
private ReadWriteLock lock = new ReentrantReadWriteLock();
public void write(String key, String value) {
lock.writeLock().lock(); // 写锁
try {
System.out.println(Thread.currentThread().getName() + "线程开始写入");
map.put(key, value);
System.out.println(Thread.currentThread().getName() + "线程写入ok");
}finally {
lock.writeLock().unlock(); // 释放写锁
}
}
public void read(String key) {
lock.readLock().lock(); // 读锁
try {
System.out.println(Thread.currentThread().getName() + "线程开始读取");
map.get(key);
System.out.println(Thread.currentThread().getName() + "线程写读取ok");
}finally {
lock.readLock().unlock(); // 释放读锁
}
}
}
1线程开始写入
1线程写入ok
6线程开始写入
6线程写入ok
3线程开始写入
3线程写入ok
2线程开始写入
2线程写入ok
5线程开始写入
5线程写入ok
4线程开始写入
4线程写入ok
1线程开始读取
5线程开始读取
2线程开始读取
1线程写读取ok
3线程开始读取
2线程写读取ok
6线程开始读取
6线程写读取ok
5线程写读取ok
4线程开始读取
4线程写读取ok
3线程写读取ok
Process finished with exit code 0
会发现,全写完,才会进行读操作!而且读顺序无
ReadWriteLock
独占锁(写锁)一次只能被一个线程占有
共享锁(读锁)多个线程可以同时占有
读-读 可以共存!
读-写 不能共存!
写-写 不能共存!
7. 阻塞队列
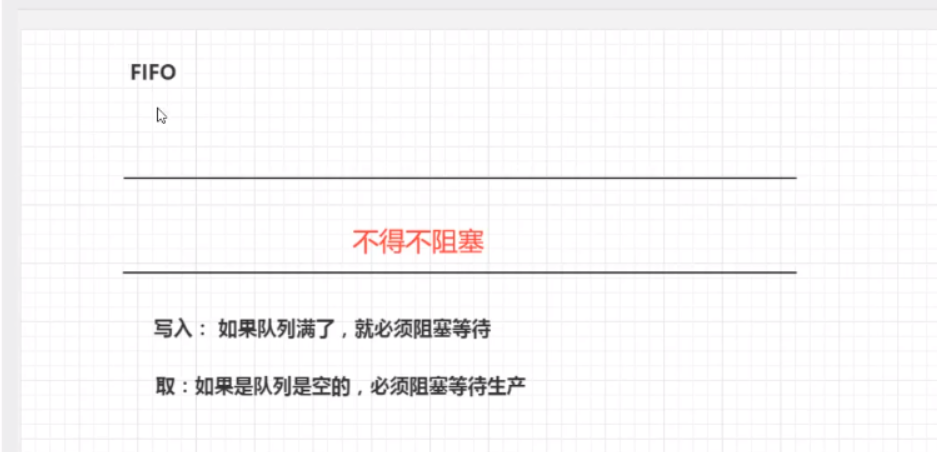
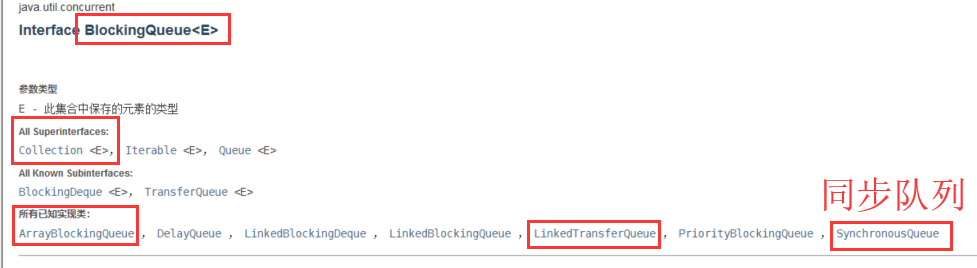
1)BlockQueue
是Collection的一个子类
什么情况下我们会使用阻塞队列
🚀 1. 生产者-消费者模型
🚀 2. 线程池的任务队列,当有新的任务到达时,他们会添加到阻塞队列中,当线程池有空闲线程时(窗口),会从队列中获取任务并执行。
🚀 3.线程同步的同步队列,一个放进去,一个才能取。
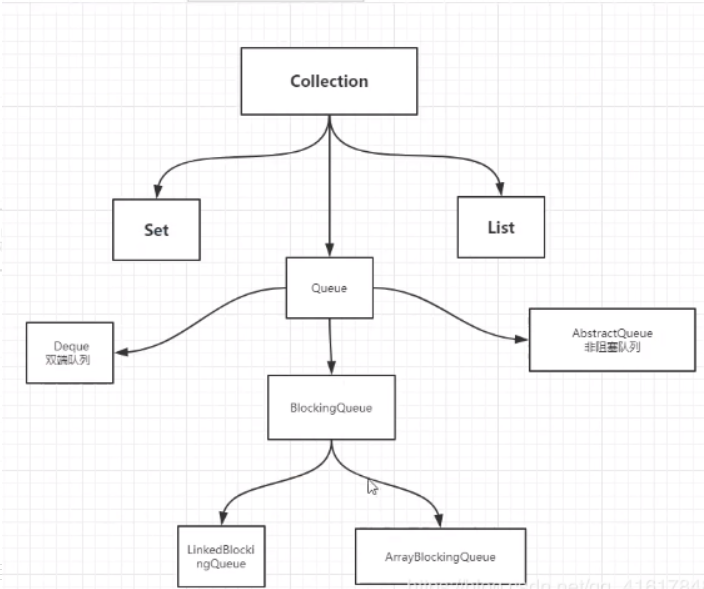
BlockingQueue 有四组api

/**
* 抛出异常
*/
public static void test1(){
//需要初始化队列的大小
ArrayBlockingQueue blockingQueue = new ArrayBlockingQueue<>(3);
System.out.println(blockingQueue.add("a"));
System.out.println(blockingQueue.add("b"));
System.out.println(blockingQueue.add("c"));
//抛出异常:java.lang.IllegalStateException: Queue full
// System.out.println(blockingQueue.add("d"));
System.out.println(blockingQueue.remove());
System.out.println(blockingQueue.remove());
System.out.println(blockingQueue.remove());
//如果多移除一个
//这也会造成 java.util.NoSuchElementException 抛出异常
System.out.println(blockingQueue.remove());
}
=======================================================================================
/**
* 不抛出异常,有返回值
*/
public static void test2(){
ArrayBlockingQueue blockingQueue = new ArrayBlockingQueue<>(3);
System.out.println(blockingQueue.offer("a"));
System.out.println(blockingQueue.offer("b"));
System.out.println(blockingQueue.offer("c"));
//添加 一个不能添加的元素 使用offer只会返回false 不会抛出异常
System.out.println(blockingQueue.offer("d"));
System.out.println(blockingQueue.poll());
System.out.println(blockingQueue.poll());
System.out.println(blockingQueue.poll());
//弹出 如果没有元素 只会返回null 不会抛出异常
System.out.println(blockingQueue.poll());
}
=======================================================================================
/**
* 等待 一直阻塞
*/
public static void test3() throws InterruptedException {
ArrayBlockingQueue blockingQueue = new ArrayBlockingQueue<>(3);
//一直阻塞 不会返回
blockingQueue.put("a");
blockingQueue.put("b");
blockingQueue.put("c");
//如果队列已经满了, 再进去一个元素 这种情况会一直等待这个队列 什么时候有了位置再进去,程序不会停止
// blockingQueue.put("d");
System.out.println(blockingQueue.take());
System.out.println(blockingQueue.take());
System.out.println(blockingQueue.take());
//如果我们再来一个 这种情况也会等待,程序会一直运行 阻塞
System.out.println(blockingQueue.take());
}
=======================================================================================
/**
* 等待 超时阻塞
* 这种情况也会等待队列有位置 或者有产品 但是会超时结束
*/
public static void test4() throws InterruptedException {
ArrayBlockingQueue blockingQueue = new ArrayBlockingQueue<>(3);
blockingQueue.offer("a");
blockingQueue.offer("b");
blockingQueue.offer("c");
System.out.println("开始等待");
blockingQueue.offer("d",2, TimeUnit.SECONDS); //超时时间2s 等待如果超过2s就结束等待
System.out.println("结束等待");
System.out.println("===========取值==================");
System.out.println(blockingQueue.poll());
System.out.println(blockingQueue.poll());
System.out.println(blockingQueue.poll());
System.out.println("开始等待");
blockingQueue.poll(2,TimeUnit.SECONDS); //超过两秒 我们就不要等待了
System.out.println("结束等待");
}
2)同步队列(SynchronousQueue)
同步队列 没有容量,也可以视为容量为1的队列;
进去一个元素,必须等待取出来之后,才能再往里面放入一个元素;
put方法 和 take方法;
Synchronized 和 其他的BlockingQueue 不一样 它不存储元素;
put了一个元素,就必须从里面先take出来,否则不能再put进去值!
并且SynchronousQueue 的take是使用了lock锁保证线程安全的。
public class SynchronousQueue {
public static void main(String[] args) {
BlockingQueue<String> synchronousQueue = new java.util.concurrent.SynchronousQueue<>();
// 网queue中添加元素
new Thread(() -> {
try {
System.out.println(Thread.currentThread().getName() + "put 01");
synchronousQueue.put("1");
System.out.println(Thread.currentThread().getName() + "put 02");
synchronousQueue.put("2");
System.out.println(Thread.currentThread().getName() + "put 03");
synchronousQueue.put("3");
} catch (InterruptedException e) {
e.printStackTrace();
}
}).start();
// 取出元素
new Thread(()-> {
try {
System.out.println(Thread.currentThread().getName() + "take" + synchronousQueue.take());
System.out.println(Thread.currentThread().getName() + "take" + synchronousQueue.take());
System.out.println(Thread.currentThread().getName() + "take" + synchronousQueue.take());
}catch (InterruptedException e) {
e.printStackTrace();
}
}).start();
}
}
Thread-0:put 01
Thread-1:take1
Thread-0:put 02
Thread-1:take2
Thread-0:put 03
Thread-1:take3
Process finished with exit code 0
3)手写一个阻塞队列
- 锁机制:使用
ReentrantLock
来保证线程安全。 - 条件变量:使用
Condition
来实现阻塞和唤醒机制。
import java.util.LinkedList;
import java.util.Queue;
import java.util.concurrent.locks.Condition;
import java.util.concurrent.locks.ReentrantLock;
public class SimpleBlockingQueue<T> {
private final Queue<T> queue = new LinkedList<>(); // 使用LinkedList作为底层队列
private final int capacity; // 队列容量
private final ReentrantLock lock = new ReentrantLock(); // 锁
private final Condition notEmpty = lock.newCondition(); // 非空条件
private final Condition notFull = lock.newCondition(); // 非满条件
public SimpleBlockingQueue(int capacity) {
this.capacity = capacity;
}
// 添加元素(阻塞)
public void put(T item) throws InterruptedException {
lock.lock();
try {
while (queue.size() == capacity) {
notFull.await(); // 队列已满,等待
}
queue.add(item);
notEmpty.signal(); // 唤醒消费者
} finally {
lock.unlock();
}
}
// 移除元素(阻塞)
public T take() throws InterruptedException {
lock.lock();
try {
while (queue.isEmpty()) {
notEmpty.await(); // 队列为空,等待
}
T item = queue.poll();
notFull.signal(); // 唤醒生产者
return item;
} finally {
lock.unlock();
}
}
// 获取队列大小
public int size() {
lock.lock();
try {
return queue.size();
} finally {
lock.unlock();
}
}
}
测试代码
public class SimpleBlockingQueueTest {
public static void main(String[] args) {
SimpleBlockingQueue<Integer> queue = new SimpleBlockingQueue<>(5);
// 生产者线程
Thread producer = new Thread(() -> {
try {
for (int i = 0; i < 10; i++) {
queue.put(i);
System.out.println("Produced: " + i);
}
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
});
// 消费者线程
Thread consumer = new Thread(() -> {
try {
for (int i = 0; i < 10; i++) {
int value = queue.take();
System.out.println("Consumed: " + value);
}
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
});
producer.start();
consumer.start();
}
}
8. 线程池(重要)
线程池:三大方式、七大参数、四种拒绝策略
程序的运行,本质:占用系统的资源!我们需要去优化资源的使用 ===> 池化技术
线程池、JDBC的连接池、内存池、对象池 等等。。。。
资源的创建、销毁十分消耗资源
池化技术:事先准备好一些资源,如果有人要用,就来我这里拿,用完之后还给我,以此来提高效率。
1)线程池的好处:
1、降低资源的消耗(频繁创建销毁);
2、提高响应的速度;
3、方便管理;
线程复用、可以控制最大并发数、管理线程;
阿里巴巴手册规约:
【强制】线程池不允许使用Executors
去创建,而是通过ThreadPoolExecutor
的方式,这样的处理方式让写的同学更加明确线程池的运行规则规避资源耗尽的风险。 说明:Executors
返回的线程池对象的弊端如下: FixedThreadPool
和 SingleThreadPool
: 允许的请求队列长度为Integer.MAX_VALUE
(约为21亿),可能会堆积大量的请求,从而导致OOM。 CachedThreadPool
和 ScheduledThreadPool
: 允许的创建线程数量为Integer.MAX_VALUE
,可能会创建大量的线程,从而导致OOM。
2)线程池:三大方法
- ExecutorService threadPool = Executors.newSingleThreadExecutor();//单个线程
- ExecutorService threadPool2 = Executors.newFixedThreadPool(5); //创建一个固定的线程池的大小
- ExecutorService threadPool3 = Executors.newCachedThreadPool(); //可伸缩的
//工具类 Executors 三大方法;
public class Demo01 {
public static void main(String[] args) {
ExecutorService threadPool = Executors.newSingleThreadExecutor();//单个线程
ExecutorService threadPool2 = Executors.newFixedThreadPool(5); //创建一个固定的线程池的大小
ExecutorService threadPool3 = Executors.newCachedThreadPool(); //可伸缩的
//线程池用完必须要关闭线程池
try {
for (int i = 1; i <=100 ; i++) {
//通过线程池创建线程
threadPool.execute(()->{
System.out.println(Thread.currentThread().getName()+ " ok");
});
}
} catch (Exception e) {
e.printStackTrace();
} finally {
threadPool.shutdown();
}
}
}
3)七大参数
newSingleThreadExecutor()源码分析
/**
* Creates an Executor that uses a single worker thread operating
* off an unbounded queue. (Note however that if this single
* thread terminates due to a failure during execution prior to
* shutdown, a new one will take its place if needed to execute
* subsequent tasks.) Tasks are guaranteed to execute
* sequentially, and no more than one task will be active at any
* given time. Unlike the otherwise equivalent
* {@code newFixedThreadPool(1)} the returned executor is
* guaranteed not to be reconfigurable to use additional threads.
*
* @return the newly created single-threaded Executor
*/
public static ExecutorService newSingleThreadExecutor() {
return new FinalizableDelegatedExecutorService
(new ThreadPoolExecutor(1, 1,
0L, TimeUnit.MILLISECONDS,
new LinkedBlockingQueue<Runnable>()));
}
newFixedThreadPool()源码分析
/**
* Creates a thread pool that reuses a fixed number of threads
* operating off a shared unbounded queue. At any point, at most
* {@code nThreads} threads will be active processing tasks.
* If additional tasks are submitted when all threads are active,
* they will wait in the queue until a thread is available.
* If any thread terminates due to a failure during execution
* prior to shutdown, a new one will take its place if needed to
* execute subsequent tasks. The threads in the pool will exist
* until it is explicitly {@link ExecutorService#shutdown shutdown}.
*
* @param nThreads the number of threads in the pool
* @return the newly created thread pool
* @throws IllegalArgumentException if {@code nThreads <= 0}
*/
public static ExecutorService newFixedThreadPool(int nThreads) {
return new ThreadPoolExecutor(nThreads, nThreads,
0L, TimeUnit.MILLISECONDS,
new LinkedBlockingQueue<Runnable>());
}
newCachedThreadPool()源码分析
/**
* Creates a thread pool that creates new threads as needed, but
* will reuse previously constructed threads when they are
* available. These pools will typically improve the performance
* of programs that execute many short-lived asynchronous tasks.
* Calls to {@code execute} will reuse previously constructed
* threads if available. If no existing thread is available, a new
* thread will be created and added to the pool. Threads that have
* not been used for sixty seconds are terminated and removed from
* the cache. Thus, a pool that remains idle for long enough will
* not consume any resources. Note that pools with similar
* properties but different details (for example, timeout parameters)
* may be created using {@link ThreadPoolExecutor} constructors.
*
* @return the newly created thread pool
*/
public static ExecutorService newCachedThreadPool() {
return new ThreadPoolExecutor(0, Integer.MAX_VALUE,
60L, TimeUnit.SECONDS,
new SynchronousQueue<Runnable>());
}
可以看到,三个方法的底层都是new ThreadPoolExecutor
public ThreadPoolExecutor(int corePoolSize, //核心线程池大小
int maximumPoolSize, //最大的线程池大小
long keepAliveTime, //超时了没有人调用就会释放
TimeUnit unit, //超时单位
BlockingQueue<Runnable> workQueue, //阻塞队列
ThreadFactory threadFactory, //线程工厂 创建线程的 一般不用动
RejectedExecutionHandler handler //拒绝策略
) {
if (corePoolSize < 0 ||
maximumPoolSize <= 0 ||
maximumPoolSize < corePoolSize ||
keepAliveTime < 0)
throw new IllegalArgumentException();
if (workQueue == null || threadFactory == null || handler == null)
throw new NullPointerException();
this.corePoolSize = corePoolSize;
this.maximumPoolSize = maximumPoolSize;
this.workQueue = workQueue;
this.keepAliveTime = unit.toNanos(keepAliveTime);
this.threadFactory = threadFactory;
this.handler = handler;
}
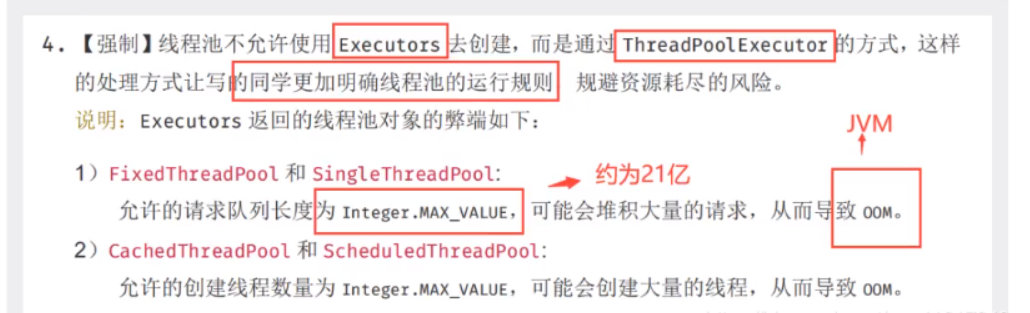
阿里巴巴的Java操作手册中明确说明:对于Integer.MAX_VALUE初始值较大,所以一般情况我们要使用底层的ThreadPoolExecutor来创建线程池。
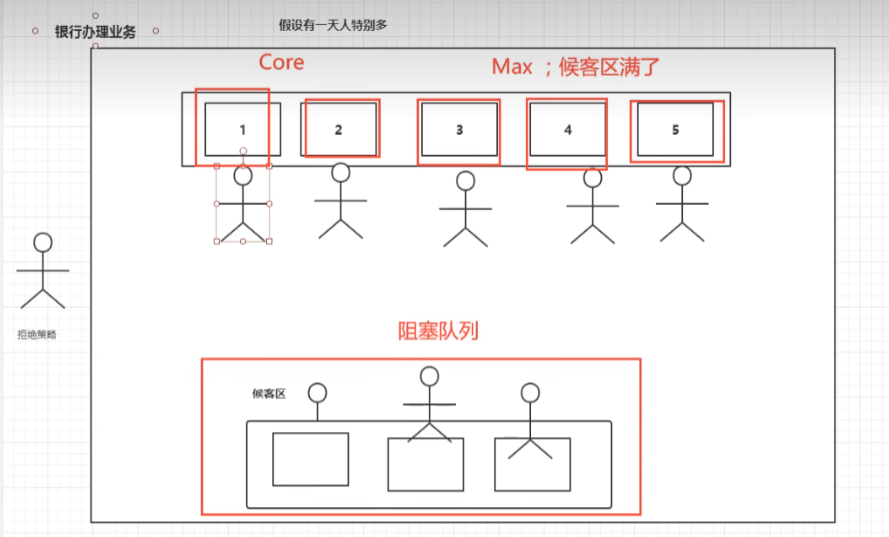
模拟上面的银行业务
核心线程大小设为2:就是一直工作的窗口
最大线程设为5:就是银行最多的工作窗口
keepAliveTime设置为1小时:如果1小时都没有业务,就关闭窗口
候客区:new LinkedBlockingQueue(3),假设候客区最多3个人
线程工厂:就用默认的,Executors.defaultThreaFactory()
拒绝策略: 可以发现有4种拒绝策略,用默认的AbortPolicy()//银行满了,但是还有人进来,就不处理这个人,并抛出异常
提示
配置时需要根据业务场景进行调整,比如对于CPU密集型任务,corePoolSize
可以设置为CPU核心数 + 1
,而对于I/O密集型任务,可以设置更大的线程数。
public class PollDemo {
public static void main(String[] args) {
// 获取cpu 的核数
int max = Runtime.getRuntime().availableProcessors();
ExecutorService service =new ThreadPoolExecutor(
2,
max,
3,
TimeUnit.SECONDS,
new LinkedBlockingDeque<>(3),
Executors.defaultThreadFactory(),
new ThreadPoolExecutor.AbortPolicy()
);
try {
for (int i = 1; i <= 10; i++) {
service.execute(() -> {
System.out.println(Thread.currentThread().getName() + "ok");
});
}
}catch (Exception e) {
e.printStackTrace();
}
finally {
service.shutdown();
}
}
}
输出结果:
//5:两个在办理,三个在等候区
pool-1-thread-1:ok
pool-1-thread-2:ok
pool-1-thread-1:ok
pool-1-thread-2:ok
pool-1-thread-1:ok
//6:6个人队列(3个)会满,所以新起1个线程,总共3个线程
pool-1-thread-2:ok
pool-1-thread-2:ok
pool-1-thread-1:ok
pool-1-thread-3:ok
pool-1-thread-2:ok
pool-1-thread-1:ok
//7:6个人队列(3个)会满,所以新起2个线程,总共4个线程
//8:6个人队列(3个)会满,所以新起3个线程,总共5个线程,达到最大了!
//9:抛出拒绝异常
4)四种拒绝策略
new ThreadPoolExecutor.AbortPolicy()
: //该拒绝策略为:银行满了,还有人进来,不处理这个人的,并抛出异常
超出最大承载,就会抛出异常:队列容量大小+maxPoolSize
new ThreadPoolExecutor.CallerRunsPolicy()
: //该拒绝策略为:哪来的去哪里 main线程进行处理pool-1-thread-1:ok main:ok pool-1-thread-1:ok pool-1-thread-2:ok pool-1-thread-1:ok pool-1-thread-5:ok pool-1-thread-4:ok pool-1-thread-3:ok pool-1-thread-2:ok
new ThreadPoolExecutor.DiscardPolicy()
: //该拒绝策略为:队列满了,丢掉异常,不会抛出异常。new ThreadPoolExecutor.DiscardOldestPolicy()
://该拒绝策略为:队列满了,尝试去和最早的进程竞争,不会抛出异常Disconnected from the target VM, address: '127.0.0.1:51839', transport: 'socket'
5)如何设置线程池的大小
1、CPU密集型(比如找出1-1000000中的素数):电脑的核数是几就选择几再+1;
只不过,为了应对线程执行过程发生缺页中断或其他异常导致线程阻塞的请求,我们可以额外在多设置一个线程,这样当某个线程暂时不需要CPU时,可以有替补线程来继续充分利用CPU。
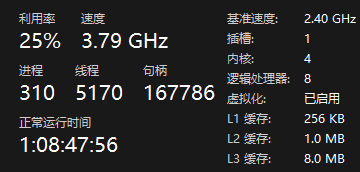
可以通过以下AP拿到你电脑的核心数:
Runtime.getRuntime().availableProcessors()
// 获取cpu 的核数
int max = Runtime.getRuntime().availableProcessors();
ExecutorService service =new ThreadPoolExecutor(
2,
max,
3,
TimeUnit.SECONDS,
new LinkedBlockingDeque<>(3),
Executors.defaultThreadFactory(),
new ThreadPoolExecutor.AbortPolicy()
);
2、I/O密集型(比如文件1O、网络1O):
我们在来看1O型任务,线程在执行IO型任务时,可能大部分时间都阻塞在10上,假如现在有10个CPU,如果我们只设置了10个线程来执行1O型任务,那么很有可能这10个线程都阻塞在了IO上,这样这10个CPU就都没活干了,所以,对于IO型任务,我们通常会设置线程数为:2*CPU核心数。
9. 四大函数式接口
新时代的程序员:lambda表达 式、链式编程、函数式接口、Stream流式计算
函数式接口:只有一个方法的接口
简化编程模型,在新版本的框架底层大量应用
foreach(消费者类型的函数式接口)
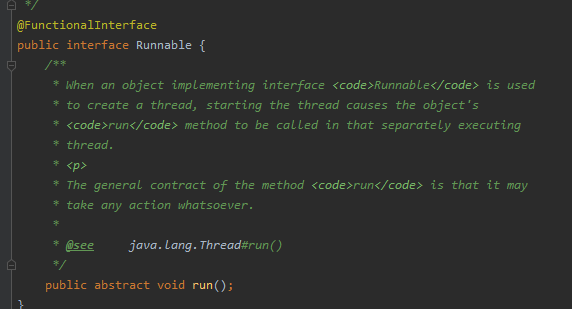
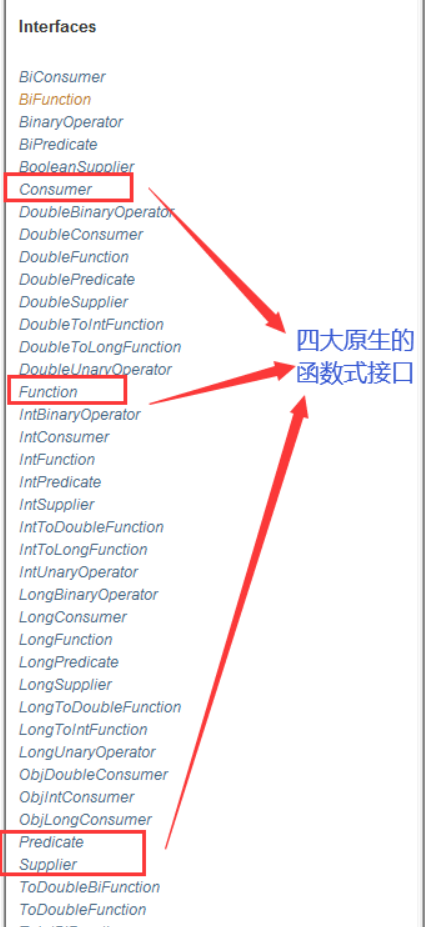
1)Function 函数型接口
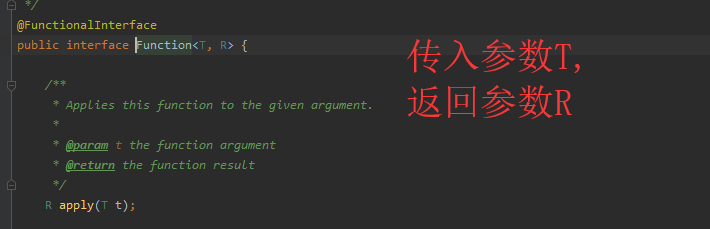
//Function函数型接口,有一个输入参数,有一个输出
//只要是函数型接口可以用Lambda表达式简化
public class Demo1{
public static void main(String[] args) {
Function function = new Function<String,String>() {
@Override
public String apply(String str) {
return str;
}
};
System.out.println(function.apply("asd"));
}
}
public class FunctionDemo {
public static void main(String[] args) {
Function<String, String> function = (str) -> {return str;};
System.out.println(function.apply("aaaaaaaaaa"));
}
}
2)Predicate 断定型接口
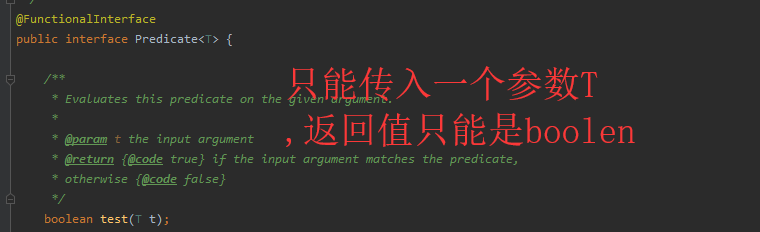
//断定型接口:有一个输入参数,
public class Demo02{
public static void main(String[]args) {
//判断字符串是否为空
Predicate<String> predicate = new Predicate<String>() {
@Override
public boolean test(String str) {
return str.isEmpty();
}
};
System.out.println(predicate.test("hello"));
}
}
public class PredicateDemo {
public static void main(String[] args) {
Predicate<String> predicate = (str) -> {return str.isEmpty();};
// false
System.out.println(predicate.test("aaa"));
// true
System.out.println(predicate.test(""));
}
}
3)Suppier 供给型接口
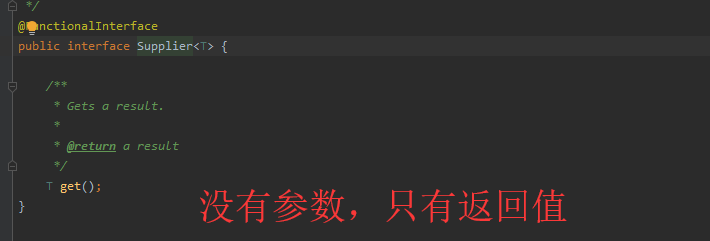
/**
* 供给型接口,只返回,不输入
*/
public class Demo3 {
public static void main(String[] args) {
Supplier<Integer> supplier = new Supplier<Integer>() {
@Override
public Integer get() {
System.out.println("get()");
return 1024;
}
};
Supplier supplier2 = ()->{return "1024";};
System.out.println(supplier2.get());
}
}
/**
* 供给型接口,只返回,不输入
*/
public class Demo4 {
public static void main(String[] args) {
Supplier<String> supplier = ()->{return "1024";};
System.out.println(supplier.get());
}
}
4)Consummer 消费型接口
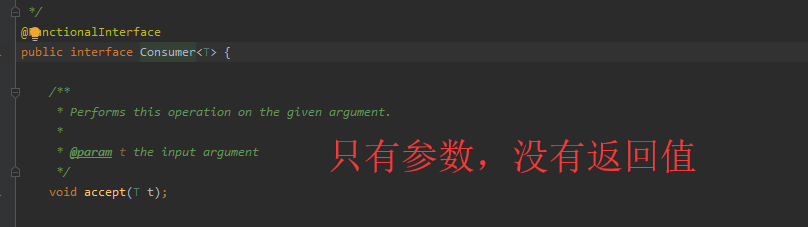
public class Demo4 {
public static void main(String[] args) {
Consumer<String> consumer = new Consumer<String>() {
@Override
public void accept(String str) {
System.out.println(str);
}
};
consumer.accept("sad");
}
}
/**
* 消费型接口 没有返回值!只有输入!
*/
public class Demo4 {
public static void main(String[] args) {
Consumer<String> consumer = (str)->{
System.out.println(str);
};
consumer.accept("abc");
}
}
10. Stream 流式计算
什么啊Stream流式计算
大数据:存储+计算
集合、Mysql本质计算存储东西的
计算都应该交给流来操作
/**
* Description:
* 题目要求: 用一行代码实现
* 1. Id 必须是偶数
* 2.年龄必须大于23
* 3. 用户名转为大写
* 4. 用户名倒序
* 5. 只能输出一个用户
*
* @author jiaoqianjin
* Date: 2020/8/12 14:55
**/
public class StreamDemo {
public static void main(String[] args) {
User u1 = new User(1, "a", 23);
User u2 = new User(2, "b", 23);
User u3 = new User(3, "c", 23);
User u4 = new User(6, "d", 24);
User u5 = new User(4, "e", 25);
List<User> list = Arrays.asList(u1, u2, u3, u4, u5);
// lambda、链式编程、函数式接口、流式计算
list.stream()
.filter(user -> {return user.getId()%2 == 0;})
.filter(user -> {return user.getAge() > 23;})
.map(user -> {return user.getName().toUpperCase();})
.sorted((user1, user2) -> {return user2.compareTo(user1);})
.limit(1)
.forEach(System.out::println);
}
}
11. ForkJoin(分支合并)
ForkJoin 在JDK1.7,并行执行任务!提高效率~。在大数据量速率会更快!
大数据中:MapReduce 核心思想->把大任务拆分为小任务!
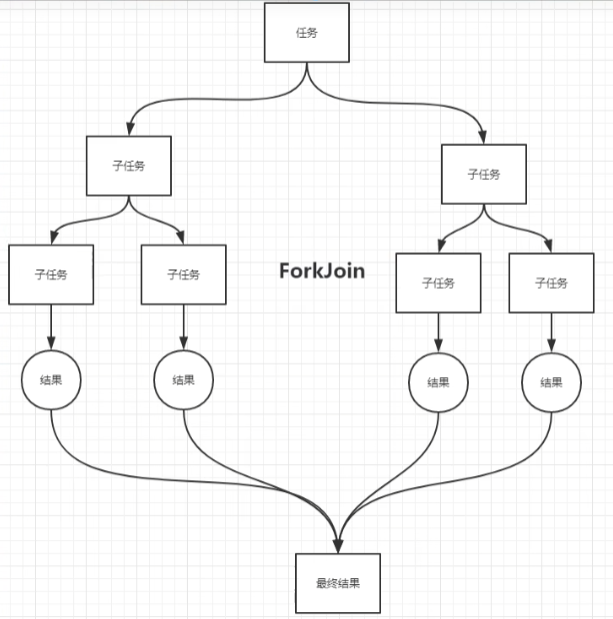
1)ForkJoin 特点: 工作窃取!
实现原理是:双端队列!从上面和下面都可以去拿到任务进行执行!
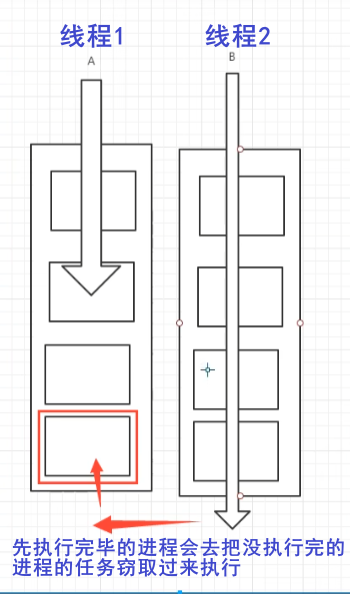
2)如何使用ForkJoin?
1、通过ForkJoinPool来执行
2、计算任务 execute(ForkJoinTask<?> task)
3、计算类要去继承ForkJoinTask;
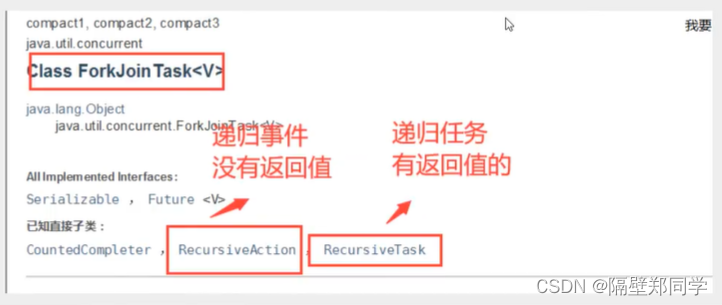
ForkJoin 的计算类
public class ForkJoinDemo extends RecursiveTask<Long> {
private long star;
private long end;
/** 临界值 */
private long temp = 1000000L;
public ForkJoinDemo(long star, long end) {
this.star = star;
this.end = end;
}
/**
* 计算方法
* @return
*/
@Override
protected Long compute() {
if ((end - star) < temp) {
Long sum = 0L;
for (Long i = star; i < end; i++) {
sum += i;
}
return sum;
}else {
// 使用ForkJoin 分而治之 计算
//1 . 计算平均值
long middle = (star + end) / 2;
ForkJoinDemo forkJoinDemo1 = new ForkJoinDemo(star, middle);
// 拆分任务,把线程压入线程队列
forkJoinDemo1.fork();
ForkJoinDemo forkJoinDemo2 = new ForkJoinDemo(middle+1,end);
forkJoinDemo2.fork();
long taskSum = forkJoinDemo1.join() + forkJoinDemo2.join();
return taskSum;
}
}
}
测试类
public class ForkJoinTest {
private static final long SUM = 20_0000_0000;
public static void main(String[] args) throws ExecutionException, InterruptedException {
test1();
test2();
test3();
}
/**
* 使用普通方法
*/
public static void test1() {
long star = System.currentTimeMillis();
long sum = 0L;
for (long i = 1; i < SUM ; i++) {
sum += i;
}
long end = System.currentTimeMillis();
System.out.println(sum);
System.out.println("时间:" + (end - star));
System.out.println("----------------------");
}
/**
* 使用ForkJoin 方法
*/
public static void test2() throws ExecutionException, InterruptedException {
long star = System.currentTimeMillis();
ForkJoinPool forkJoinPool = new ForkJoinPool();
ForkJoinTask<Long> task = new ForkJoinDemo(0L, SUM);
ForkJoinTask<Long> submit = forkJoinPool.submit(task);
Long along = submit.get();
System.out.println(along);
long end = System.currentTimeMillis();
System.out.println("时间:" + (end - star));
System.out.println("-----------");
}
/**
* 使用 Stream 流计算
*/
public static void test3() {
long star = System.currentTimeMillis();
long sum = LongStream.range(0L, 20_0000_0000L).parallel().reduce(0, Long::sum);
System.out.println(sum);
long end = System.currentTimeMillis();
System.out.println("时间:" + (end - star));
System.out.println("-----------");
}
}
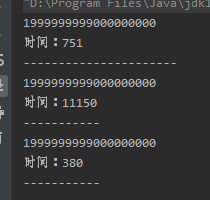
.parallel().reduce(0, Long::sum)使用一个并行流去计算整个计算,提高效率。
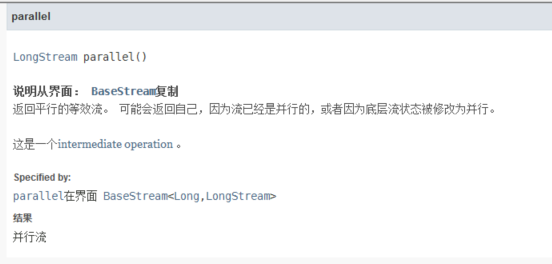
12. 异步回调
Future 设计的初衷:对将来的某个事件结果进行建模!
其实就是前端 --> 发送ajax异步请求给后端
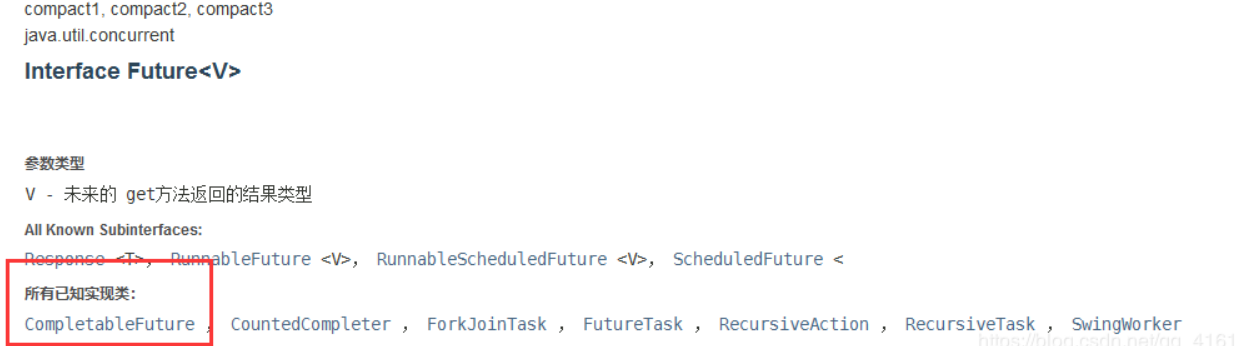
但是我们平时都使用CompletableFuture
(1)没有返回值的runAsync异步回调
public static void main(String[] args) throws ExecutionException, InterruptedException
{
// 发起 一个 请求
System.out.println(System.currentTimeMillis());
System.out.println("---------------------");
CompletableFuture<Void> future = CompletableFuture.runAsync(()->{
//发起一个异步任务
try {
TimeUnit.SECONDS.sleep(2);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(Thread.currentThread().getName()+".....");
});
System.out.println(System.currentTimeMillis());
System.out.println("------------------------------");
//输出执行结果
System.out.println(future.get()); //获取执行结果
}
(2)有返回值的异步回调supplyAsync
//有返回值的异步回调
CompletableFuture<Integer> completableFuture=CompletableFuture.supplyAsync(()->{
System.out.println(Thread.currentThread().getName());
try {
TimeUnit.SECONDS.sleep(2);
int i=1/0;
} catch (InterruptedException e) {
e.printStackTrace();
}
return 1024;
});
System.out.println(completableFuture.whenComplete((t, u) -> {
//success 回调
System.out.println("t=>" + t); //正常的返回结果
System.out.println("u=>" + u); //抛出异常的 错误信息
}).exceptionally((e) -> {
//error回调
System.out.println(e.getMessage());
return 404;
}).get());
whenComplete: 有两个参数,一个是t 一个是u
T:是代表的 正常返回的结果;
U:是代表的 抛出异常的错误信息;
如果发生了异常,get可以获取到exceptionally返回的值;
13. JMM
1)对Volatile 的理解
Volatile 是 Java 虚拟机提供 轻量级的同步机制
1、保证可见性
2、不保证原子性
3、禁止指令重排
如何实现可见性
volatile变量修饰的共享变量在进行写操作的时候回多出一行汇编:
0x01a3de1d:movb $0×0,0×1104800(%esi);0x01a3de24**:lock** addl $0×0,(%esp);
Lock前缀的指令在多核处理器下会引发两件事情。
1)将当前处理器缓存行的数据写回到系统内存。
2)这个写回内存的操作会使其他cpu里缓存了该内存地址的数据无效。
多处理器总线嗅探:
为了提高处理速度,处理器不直接和内存进行通信,而是先将系统内存的数据读到内部缓存后再进行操作,但操作不知道何时会写到内存。如果对声明了volatile的变量进行写操作,JVM就会向处理器发送一条lock前缀的指令,将这个变量所在缓存行的数据写回到系统内存。但是在多处理器下,为了保证各个处理器的缓存是一致的,就会实现缓存缓存一致性协议,每个处理器通过嗅探在总线上传播的数据来检查自己的缓存值是不是过期了,如果处理器发现自己缓存行对应的内存地址呗修改,就会将当前处理器的缓存行设置无效状态,当处理器对这个数据进行修改操作的时候,会重新从系统内存中把数据库读到处理器缓存中。
2)什么是JMM?
JMM:JAVA内存模型,不存在的东西,是一个概念,也是一个约定!
关于JMM的一些同步的约定:
1、线程解锁前,必须把共享变量立刻刷回主存;
2、线程加锁前,必须读取主存中的最新值到工作内存中;
3、加锁和解锁是同一把锁;
线程中分为 工作内存、主内存
8种操作:
- Read(读取):作用于主内存变量,它把一个变量的值从主内存传输到线程的工作内存中,以便随后的load动作使用;
- load(载入):作用于工作内存的变量,它把read操作从主存中变量放入工作内存中;
- Use(使用):作用于工作内存中的变量,它把工作内存中的变量传输给执行引擎,每当虚拟机遇到一个需要使用到变量的值,就会使用到这个指令;
- assign(赋值):作用于工作内存中的变量,它把一个从执行引擎中接受到的值放入工作内存的变量副本中;
- store(存储):作用于主内存中的变量,它把一个从工作内存中一个变量的值传送到主内存中,以便后续的write使用;
- write(写入):作用于主内存中的变量,它把store操作从工作内存中得到的变量的值放入主内存的变量中;
- lock(锁定):作用于主内存的变量,把一个变量标识为线程独占状态;
- unlock(解锁):作用于主内存的变量,它把一个处于锁定状态的变量释放出来,释放后的变量才可以被其他线程锁定;
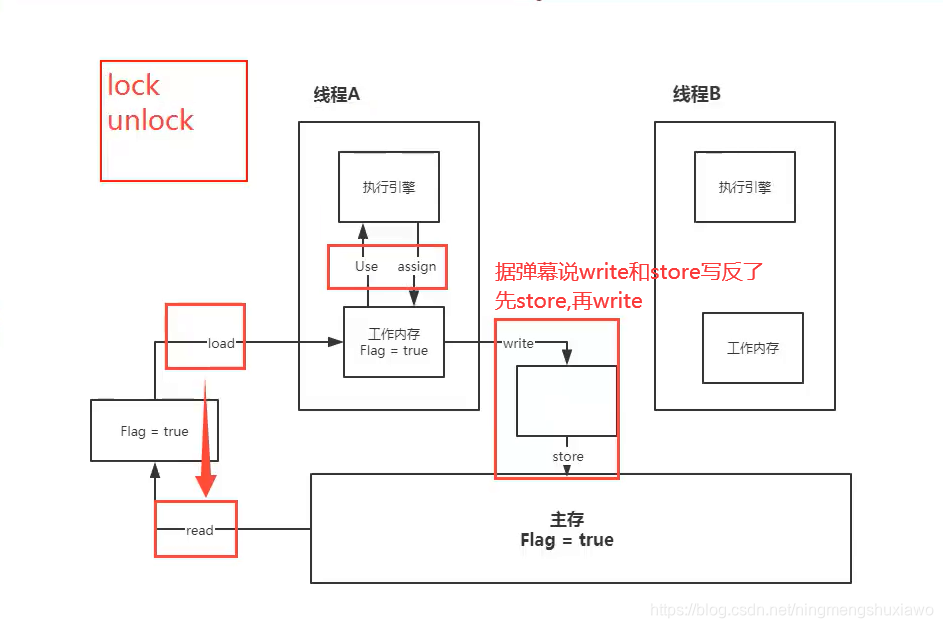
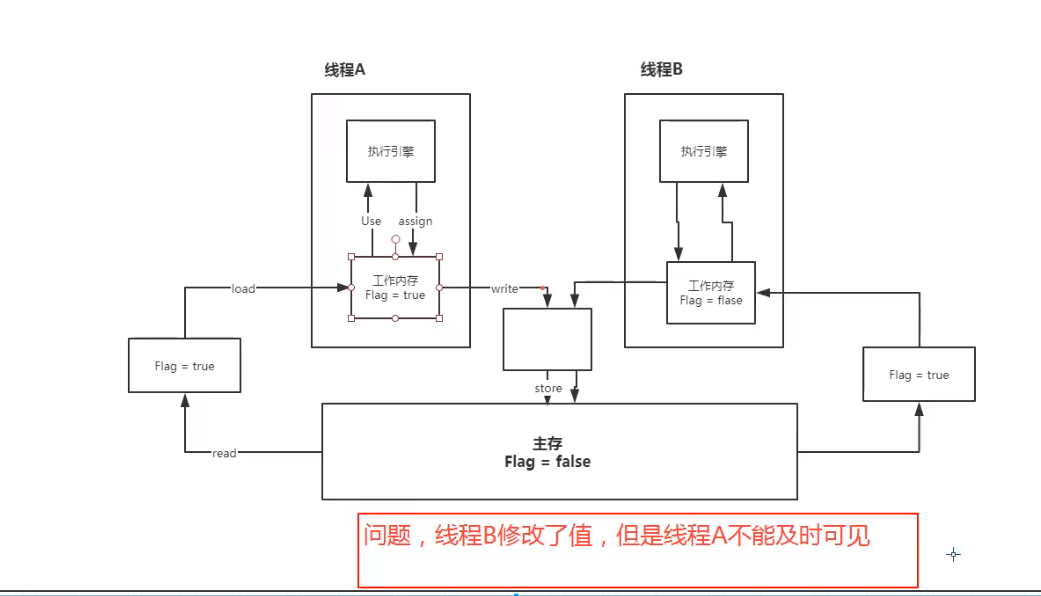
有可能会出现线程B修改了值刷新回主内存,但是线程A没有取到这个最新的值
JMM对这8种操作给了相应的规定:
- 不允许read和load、store和write操作之一单独出现。即使用了read必须load,使用了store必须write
- 不允许线程丢弃他最近的assign操作,即工作变量的数据改变了之后,必须告知主存
- 不允许一个线程将没有assign的数据从工作内存同步回主内存
- 一个新的变量必须在主内存中诞生,不允许工作内存直接使用一个未被初始化的变量。就是对变量实施use、store操作之前,必须经过assign和load操作
- 一个变量同一时间只有一个线程能对其进行lock。多次lock后,必须执行相同次数的unlock才能解锁
- 如果对一个变量进行lock操作,会清空所有工作内存中此变量的值,在执行引擎使用这个变量前,必须重新load或assign操作初始化变量的值
- 如果一个变量没有被lock,就不能对其进行unlock操作。也不能unlock一个被其他线程锁住的变量
- 对一个变量进行unlock操作之前,必须把此变量同步回主内存
14 volatile
1)保证可见性
public class JMMDemo {
// 不加 volatile 程序就会死循环!
// 加 volatile 可以保证可见性
// private volatile static int num = 0;
private static int num = 0;
public static void main(String[] args) { // main
new Thread(()->{ // 线程 1 对主内存的变化不知道的
while (num==0){
}
}).start();
try {
TimeUnit.SECONDS.sleep(1);
} catch (InterruptedException e) {
e.printStackTrace();
}
num = 1;
System.out.println(num);
}
}
但是如果在while循环里面添加了打印i的语句
while(num==0)
{
System.out.println(num);
}
程序运行一会就停止了
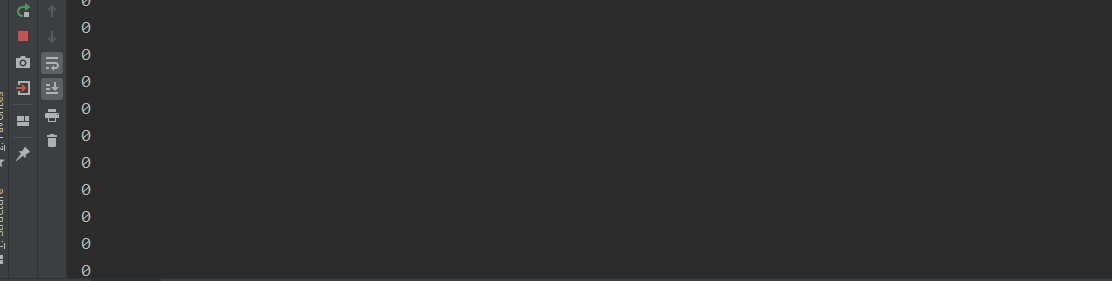
这是因为子线程强制读了一遍i的值
在循环体中打印输出语句时,println方法中有synchronized同步代码块,而synchronized也能保证对变量的修改可见性,对变量num进行操作以后,num的值也会立即刷回主存
在子线程加了输出语句会导致run方法里面的循环每输出一次进行一次判断,当内存数据修改,等缓存一致性协议生效 再判断 就会退出循环
2)不保证原子性
原子性: 不可分割
线程A在执行任务的时候,是不能被打扰的,要么同时成功,要么同时失败
public class Vdemo02 {
private static int num=0;
public static void add()
{
num++;
}
public static void main(String[] args) {
for(int i=0;i<20;i++)
{
new Thread(()->{
for(int j=0;j<1000;j++)
{
add();
}
}).start();
}
while(Thread.activeCount()>2)//因为程序里面始终是有2个线程的,main线程和gc线程
{
Thread.yield();
//Thread.yield()是在主线程中执行的,意思是还有出路GC和main之外的其他线程在跑,主线程就让出cpu不往下执行,让出然后重新竞争cpu的执行权,有可能还是main抢到,不过这里是循环,抢到继续让出,直到只有2个线程
}
//理论上打印出来应该是20000
System.out.println(Thread.currentThread().getName()+" "+num);//肯定不能直接
}
}

解决方法
我们可以加synchronized
public synchronized static void add()
{
num++;
}
如果只是给变量加上volatile
private volatile static int num=0;
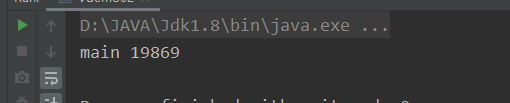
注意++不是原子操作
不加lock和synchronized,怎么保证原子性?
我们来看看对于++,jvm底层是怎么操作的
方法1:
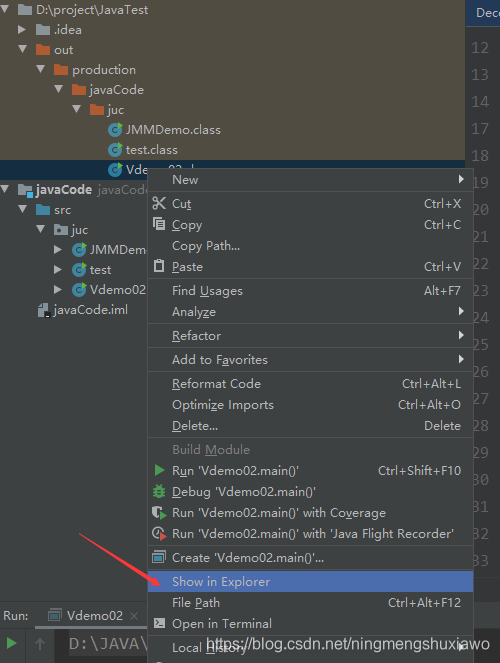
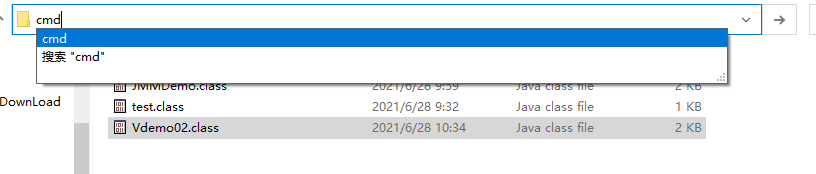
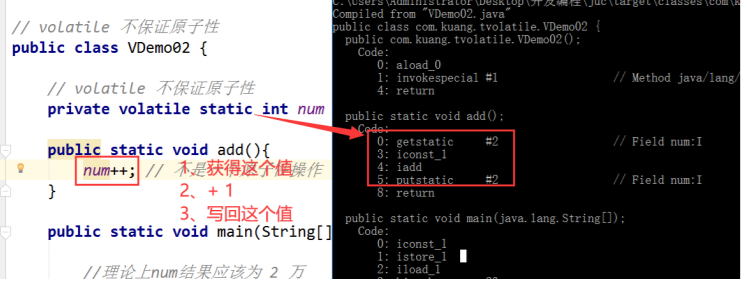
方法2:
在out目录下选中class文件,然后选择show byteCode,没有这个选项的话,你需要安装这个插件
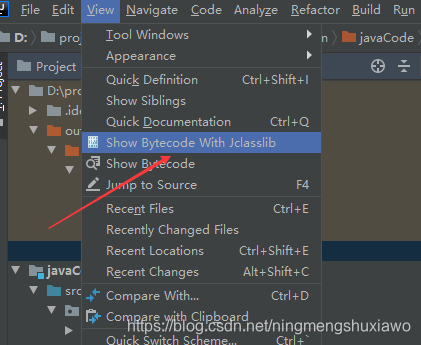
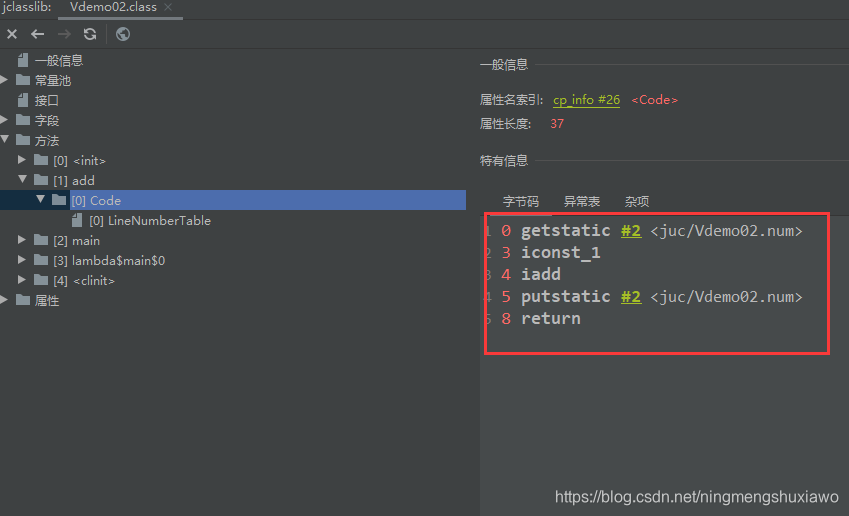
方法3:
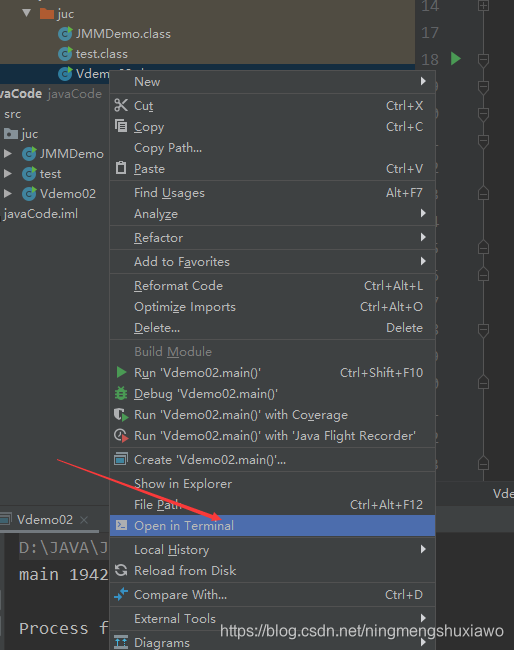

public static void add();
Code:
0: getstatic #2 // Field num:I
3: iconst_1
4: iadd
5: putstatic #2 // Field num:I
8: return
我们来分析一下++的字节码操作
第一步:获得 num的值
第二步: num的值加1
第三步: 写回num
在多线程操作的过程中,可能加1后的值还没来得及更新,就被另一个线程读到进行加1,也就是说,有一些线程用的是以前的值,所以整体加起来会小于20000
之所以小于理论值,是因为线程回写数据到主内存的时候,覆盖了已经被其他更快的线程执行的结果
假如num是0,就可能线程1加了1,还没写回,线程2就拿到了,此时num还是0,所以两个线程都操作了num,但是num的值是1,相当于只加了一次
对线程来讲只保证每次加之前,从主存取一下当前值
加的次数是固定的,有的线程取的是旧值,结果肯定小了
回到刚刚的问题,不加lock和synchronized,怎么保证原子性?
使用原子类解决原子性问题
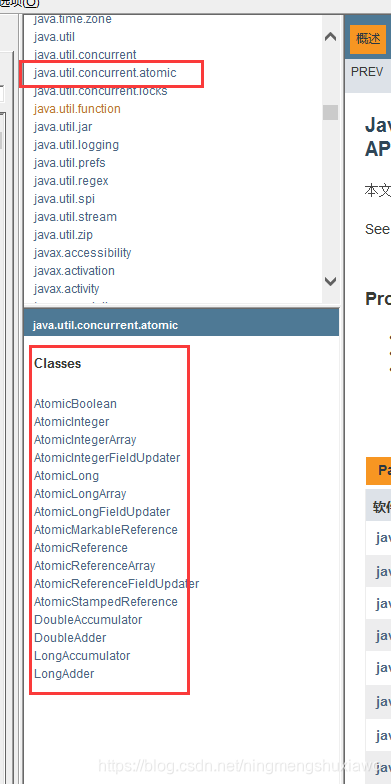
private static AtomicInteger num=new AtomicInteger();
public static void add()
{
num.getAndIncrement(); //cas
//num=num+1;
}
因为原子类底层初始化的时候,将赋值给了一个volatiel属性
原子类的底层都直接和操作系统挂钩,在内存中修改值,Unsafe类是很特殊的存在
public final int getAndIncrement() {
return unsafe.getAndAddInt(this, valueOffset, 1);
}
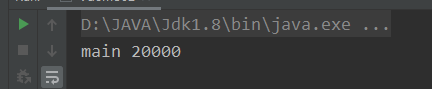
3)禁止指令重排
什么是指令重排?
我们写的程序,计算机并不是按照我们自己写的那样去执行的
源代码–>编译器优化重排–>指令并行也可能会重排–>内存系统也会重排–>执行
处理器在进行指令重排的时候,会考虑数据之间的依赖性!
int x=1; //1
int y=2; //2
x=x+5; //3
y=x*x; //4
//我们期望的执行顺序是 1_2_3_4 可能执行的顺序会变成2134 1324
//可不可能是 4123? 不可能的
可能造成的影响结果:前提:a b x y这四个值 默认都是0
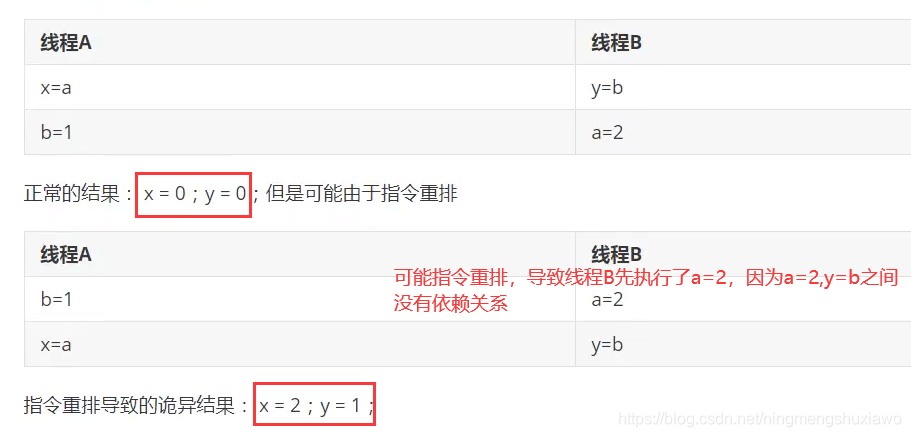
volatile可以避免指令重排:
volatile中会加一道内存的屏障,这个内存屏障可以保证在这个屏障中的指令顺序。
内存屏障:CPU指令。作用:
1、保证特定的操作的执行顺序;
2、可以保证某些变量的内存可见性(利用这些特性,就可以保证volatile实现的可见性)
volatile标注后,编译器生成代码会不优化
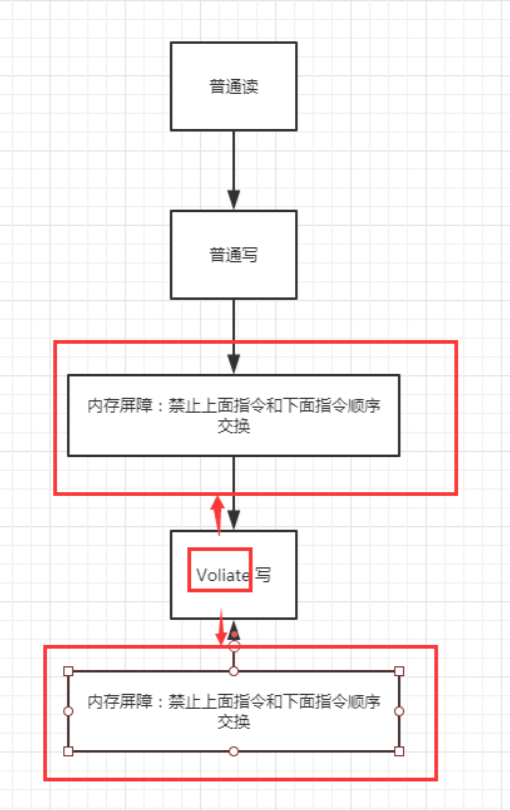
volatile写前加SS屏障写后加SL屏障,读前加LL屏障,读后加LS屏障
4)总结
- volatile可以保证可见性;
- 不能保证原子性
- 由于内存屏障,可以保证避免指令重排的现象产生
面试官:那么你知道在哪里用这个内存屏障用得最多呢?单例模式
15. 玩转单例模式
饿汉式、DCL懒汉式
说到volatile的防止指令重排,那么volatile的内存屏障在哪里使用的最多,就是单例模式了。
1)饿汉式
饿汉式的问题:可能会浪费内存
饿汉式一上来就会把所有的东西加载到内存,对象就已经存在了,对象没有使用的话,可能会浪费内存
主要特点有:
构造函数私有,避免在外部被创建对象
提前创建好对象
提供公有的接口,返回在类内部提前创建好的对象
静态变量随着类的加载就已经实例化了,跟是否调用静态方法没有关系
饿汉式加载时就会初始化,懒汉式只有在获取单例的时候才会初始化
类加载时,成员变量会被初始化,局部变量不会
/**
* 饿汉式单例
*/
public class Hungry {
/**
* 可能会浪费空间
*/
private byte[] data1=new byte[1024*1024];
private byte[] data2=new byte[1024*1024];
private byte[] data3=new byte[1024*1024];
private byte[] data4=new byte[1024*1024];
private Hungry(){
}
private final static Hungry hungry = new Hungry();
public static Hungry getInstance(){
return hungry;
}
}
2)DCL懒汉式
针对饿汉式单例的浪费内存的问题,提出了懒汉式单例,要用的时候再创建对象
public class LazyMan {
private LazyMan(){
}
private static LazyMan lazyman;//还没有创建对象,只是声明,没有new
public static LazyMan getInstance()
{
if(lazyman==null)
{
lazyman=new LazyMan();//如果这个对象为空,就实例化这个对象
}
return lazyman;
}
}
在多个线程的情况下,懒汉式单例可能会出现安全问题,就是线程1进入了if判断,并开始构造对象
public class LazyMan {
private LazyMan(){
System.out.println(Thread.currentThread().getName()+"ok");
}
private static LazyMan lazyman;//还没有创建对象,只是声明,没有new
public static LazyMan getInstance()
{
if(lazyman==null)
{
lazyman=new LazyMan();//如果这个对象为空,就实例化这个对象
}
return lazyman;
}
public static void main(String[] args) {
for (int i = 0; i < 10; i++) {
new Thread(()->{
LazyMan.getInstance();
}).start();
}
}
}
可以看到,有3个线程调用了构造函数,这说明程序中现在有3个Lazyman对象,就不是单例了,所以不安全
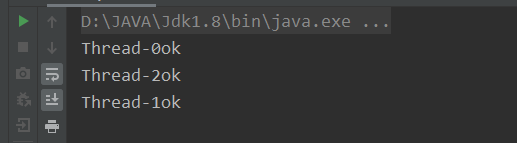
双重锁机制
public class LazyMan {
private LazyMan(){
System.out.println(Thread.currentThread().getName()+"ok");
}
private static LazyMan lazyman;//还没有创建对象,只是声明,没有new
public static LazyMan getInstance()
{
//双重检测锁模式 DCL
if(lazyman==null)
{
//如果为空,先上一层锁,锁LazyMan当前对象
synchronized (LazyMan.class){//静态方法是类锁
//如果synchronized直接写在方法上,所有线程都要抢锁,效率低,这个只有为空时才会抢锁
if(lazyman==null)//在锁里面再判断一次
{
lazyman=new LazyMan();//如果这个对象为空,就实例化这个对象
}
}
}
return lazyman;
}
public static void main(String[] args) {
for (int i = 0; i < 10; i++) {
new Thread(()->{
LazyMan.getInstance();
// System.out.println(LazyMan.getInstance());
}).start();
}
}
}
可以看到只创建了一个对象
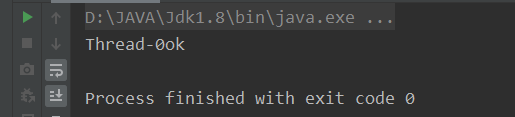
但是还是有可能出现问题
创建对象的过程在极端情况下肯定是会出现问题的,因为不是原子性操作,会经历
1 分配内存空间,
2执行构造方法(初始化对象)
3把对象指向分配的空间
但是可能会发生指令重排,可能会按132的顺序执行,就是先分配内存空间,然后用空对象先占用内存空间,占用之后再执行构造方法
如下图,很有可能A执行了13还没执行2,但是现在lazyman已经不是null了,如果现在进来一个B线程,外层判断不为空,那么B线程会直接返回lazyman,但lazyman实际上还没有完成构造,所以不安全(new只是把应用加上了,但是堆还没有创建完,return就会有问题)
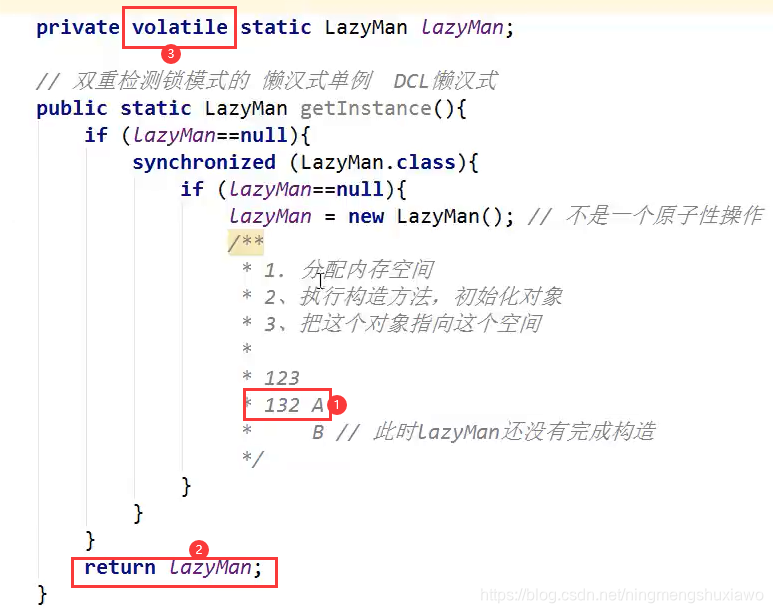
所以要用volatile修饰防止指令重排(防止第二个线程抢先执行,抢先返回一个尚未初始化完成的引用)
所以这里是同步代码块保证了操作的原子性,volatile禁止了指令重排
指令重排的原理是为了提升CPU多段流水的效率,但并不是指令任意重排,处理器必须能正确处理指令依赖关系保障程序得出正确的执行结果。
总结:synchronized保证的是if判断和new一个对象能同时成功或同时失败,但是new一个对象不是原子操作,执行13后,第二个线程认为已经new对象成功了,最上面的if判断不等于null
3)静态内部类
在一个类里面写一个静态的类
首先只要单例一定要先构造器私有
加载外部类时,不会加载静态内部类
线程安全且懒加载
但是静态内部类单例也是不安全的,因为反射可以破坏单例
//静态内部类
public class Holder {
private Holder(){
}
public static Holder getInstance(){
return InnerClass.holder;
}
public static class InnerClass{
private static final Holder holder = new Holder();
}
}
测试
public class Holder {
private Holder() {
System.out.println(Thread.currentThread().getName()+"ok");
}
public static Holder getInstance() {
return InnerClass.HOLDER;
}
public static class InnerClass {
private static final Holder HOLDER = new Holder();
}
public static void main(String[] args) {
for(int i=0;i<10;i++)
{
new Thread(()->{
Holder.getInstance();
}).start();
}
}
}
可以看到,内存中只有一个实例,就是只有一个线程进入了构造函数,因为静态类只加载一次
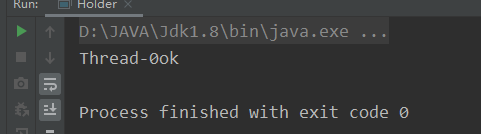
但是只要有反射,任何私有的都是纸老虎,我们以DCL的单例为例,来试试反射
public static void main(String[] args) throws NoSuchMethodException, IllegalAccessException, InvocationTargetException, InstantiationException {
// for (int i = 0; i < 10; i++) {
// new Thread(()->{
// LazyMan.getInstance();
// // System.out.println(LazyMan.getInstance());
// }).start();
// }
LazyMan instance = LazyMan.getInstance();
//获得空参构造器
Constructor<LazyMan> declaredConstructor = LazyMan.class.getDeclaredConstructor(null);
//无视私有构造器
declaredConstructor.setAccessible(true);
//通过反射创建对象
LazyMan lazyMan = declaredConstructor.newInstance();
//测试两个对象是否一样
System.out.println( instance );
System.out.println(lazyMan);
}
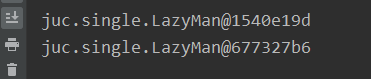
可以破解:
private LazyMan(){
synchronized (LazyMan.class){
if(lazyman!=null)
{
throw new RuntimeException("不要试图通过反射破坏单例");
}
System.out.println(Thread.currentThread().getName()+"ok");
}

看完整代码
相当于在DCL的基础上又在构造函数里面加了一重检测
public class LazyMan {
private LazyMan() {
synchronized (LazyMan.class) {
if (lazyman != null) {
throw new RuntimeException("不要试图通过反射破坏单例");
}
System.out.println(Thread.currentThread().getName() + "ok");
}
//
}
private static volatile LazyMan lazyman;//还没有创建对象,只是声明,没有new
public static LazyMan getInstance() {
//双重检测锁模式 DCL
if (lazyman == null) {
//如果为空,先上一层锁,锁LazyMan当前对象
synchronized (LazyMan.class) {//静态方法是类锁
//如果synchronized直接写在方法上,所有线程都要抢锁,效率低,这个只有为空时才会抢锁
if (lazyman == null)//在锁里面再判断一次
{
lazyman = new LazyMan();//如果这个对象为空,就实例化这个对象
}
}
}
return lazyman;
}
public static void main(String[] args) throws NoSuchMethodException, IllegalAccessException, InvocationTargetException, InstantiationException {
// for (int i = 0; i < 10; i++) {
// new Thread(()->{
// LazyMan.getInstance();
// // System.out.println(LazyMan.getInstance());
// }).start();
// }
LazyMan instance = LazyMan.getInstance();
//获得空参构造器
Constructor<LazyMan> declaredConstructor = LazyMan.class.getDeclaredConstructor(null);
//无视私有构造器
declaredConstructor.setAccessible(true);
//通过反射创建对象
LazyMan lazyMan = declaredConstructor.newInstance();
//测试两个对象是否一样
System.out.println(instance);
System.out.println(lazyMan);
}
}
现在我们不用getInstance()去获取对象,而是直接通过反射创建两个对象
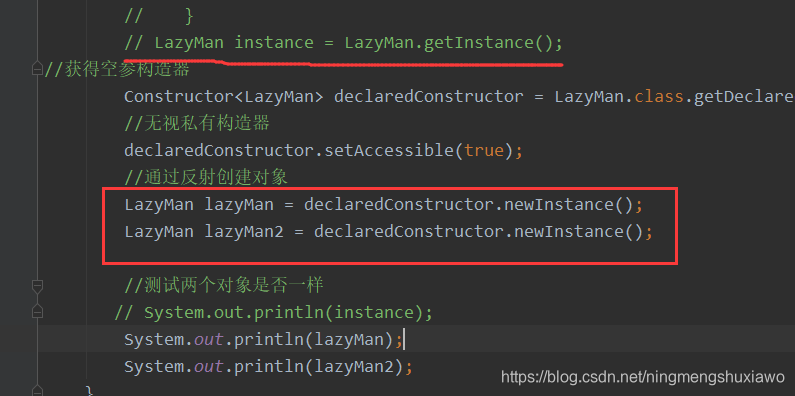
可以发现,单例又被破坏了,因为构造函数里面判断的是
if (lazyman == null)//在锁里面再判断一次
{
}
但是注意,我们用反射new 的对象跟类里面的lazyman对象肯定是不一样的啊,没有调用getInstance(),类里面的lazyman就一直为空,所以单例又被破坏了
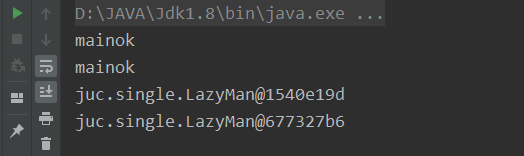
解决方法,用个标志位
private static boolean flag=false;
private LazyMan() {
synchronized (LazyMan.class) {
if( flag==false)
{
flag=true;
}
else
{
throw new RuntimeException("不要试图通过反射破坏单例");
}
}
}
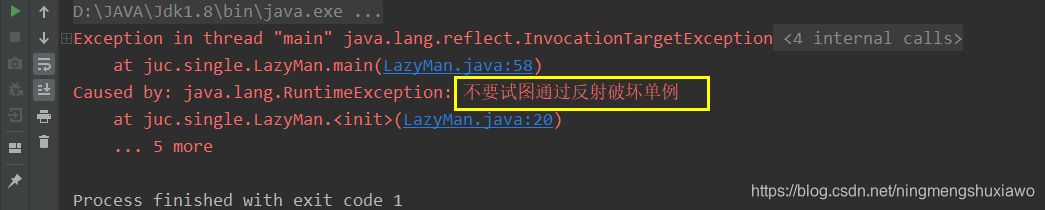
来我们继续破坏单例,我们把这个flag字段给它破坏了
Field flag=LazyMan.class.getDeclaredField("flag");
flag.setAccessible(true);
Constructor<LazyMan> declaredConstructor = LazyMan.class.getDeclaredConstructor(null);
//无视私有构造器
declaredConstructor.setAccessible(true);
//通过反射创建对象
LazyMan lazyMan = declaredConstructor.newInstance();
flag.set(lazyMan,false);//把第一个对象的flag重新改成false
LazyMan lazyMan2 = declaredConstructor.newInstance();
//测试两个对象是否一样
// System.out.println(instance);
System.out.println(lazyMan);
System.out.println(lazyMan2);
可以发现单例又被破坏了。。。。
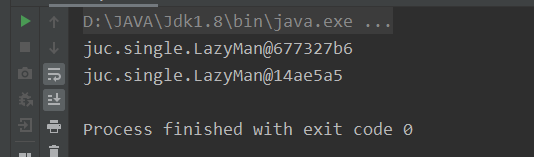
public class LazyMan {
private static boolean flag=false;
private LazyMan() {
synchronized (LazyMan.class) {
if( flag==false)
{
flag=true;
}
else
{
throw new RuntimeException("不要试图通过反射破坏单例");
}
}
}
private static volatile LazyMan lazyman;//还没有创建对象,只是声明,没有new
public static LazyMan getInstance() {
//双重检测锁模式 DCL
if (lazyman == null) {
//如果为空,先上一层锁,锁LazyMan当前对象
synchronized (LazyMan.class) {//静态方法是类锁
//如果synchronized直接写在方法上,所有线程都要抢锁,效率低,这个只有为空时才会抢锁
if (lazyman == null)//在锁里面再判断一次
{
lazyman = new LazyMan();//如果这个对象为空,就实例化这个对象
}
}
}
return lazyman;
}
public static void main(String[] args) throws NoSuchMethodException, IllegalAccessException, InvocationTargetException, InstantiationException, NoSuchFieldException {
// for (int i = 0; i < 10; i++) {
// new Thread(()->{
// LazyMan.getInstance();
// // System.out.println(LazyMan.getInstance());
// }).start();
// }
// LazyMan instance = LazyMan.getInstance();
//获得空参构造器
Field flag=LazyMan.class.getDeclaredField("flag");
flag.setAccessible(true);
Constructor<LazyMan> declaredConstructor = LazyMan.class.getDeclaredConstructor(null);
//无视私有构造器
declaredConstructor.setAccessible(true);
//通过反射创建对象
LazyMan lazyMan = declaredConstructor.newInstance();
flag.set(lazyMan,false);//把第一个对象的flag重新改成false
LazyMan lazyMan2 = declaredConstructor.newInstance();
//测试两个对象是否一样
// System.out.println(instance);
System.out.println(lazyMan);
System.out.println(lazyMan2);
}
}
那怎么解决呢?我们点进去反射的newInstance()看看呢
我们可以看到,如果类是一个枚举类型的话,就会告诉你不能使用反射破坏枚举,枚举是jdk 1.5 开始出现的,自带单例模式
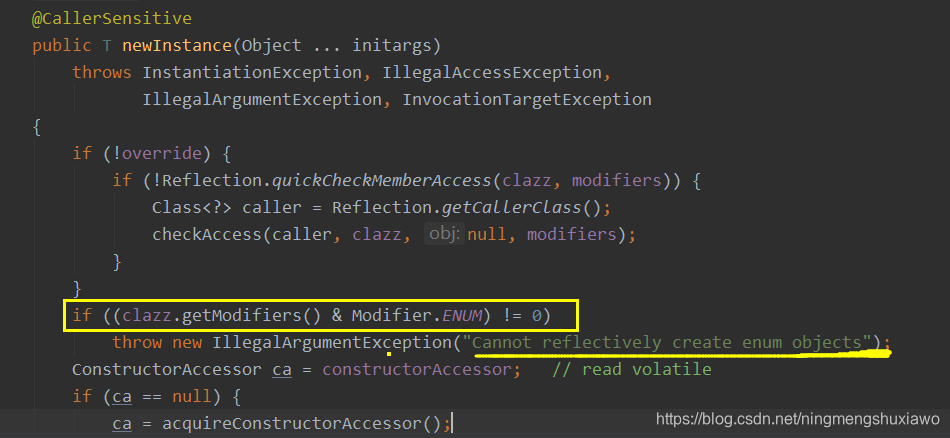
4)枚举
枚举本身也是一个类
public enum EnumSingle {
INSTANCE;
public static EnumSingle getInstance()
{
return INSTANCE;
}
}
class Test{
public static void main(String[] args) {
EnumSingle instance1=EnumSingle.INSTANCE;
EnumSingle instance2=EnumSingle.INSTANCE;
EnumSingle instance3=EnumSingle.getInstance();
System.out.println( instance1);
System.out.println( instance2);
System.out.println( instance3);
}
}
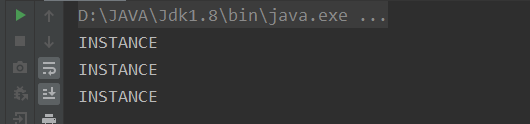
我们来试试用反射破坏枚举单例
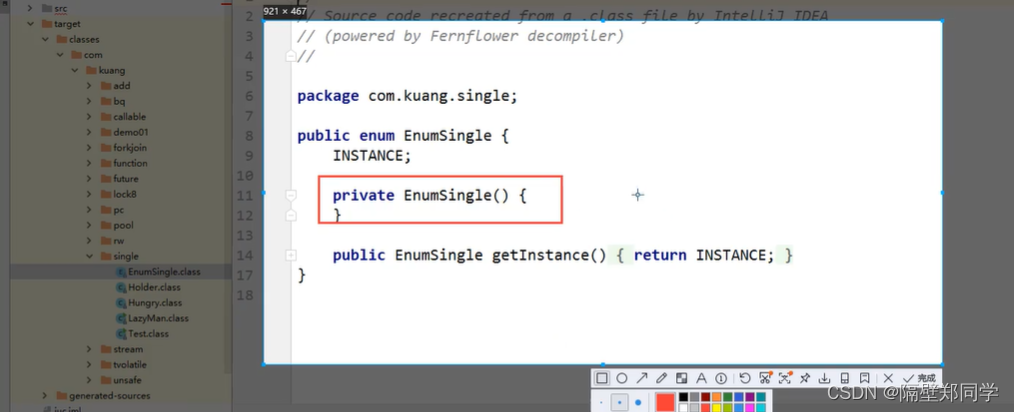
public enum EnumSingle {
INSTANCE;
public static EnumSingle getInstance()
{
return INSTANCE;
}
}
class Test{
public static void main(String[] args) throws NoSuchMethodException, IllegalAccessException, InvocationTargetException, InstantiationException {
EnumSingle instance1=EnumSingle.INSTANCE;
// EnumSingle instance2=EnumSingle.INSTANCE;
// EnumSingle instance3=EnumSingle.getInstance();
Constructor<EnumSingle> declaredConstructor = EnumSingle.class.getDeclaredConstructor(null);
declaredConstructor.setAccessible(true);//先把构造器的私有权限破除,使得反射可以访问,创建对象
EnumSingle instance2= declaredConstructor.newInstance();
System.out.println( instance1);
System.out.println( instance2);
}
}
下面的错误提示是枚举类没有空参的构造方法
也就是下面这句话出错了idea骗了我们
Constructor<EnumSingle> declaredConstructor = EnumSingle.class.getDeclaredConstructor(null);
正常破坏单例是应该报错不能使用反射破坏枚举

通过反编译我们可以看到,这个枚举本身也是一个class,它继承了一个枚举类
然而构造器还是空参的啊,说明我们还是被骗了
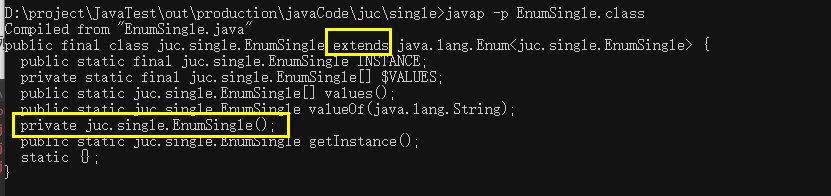
现在我们用jad.exe反编译试试
我们把class字节码生成java文件看看

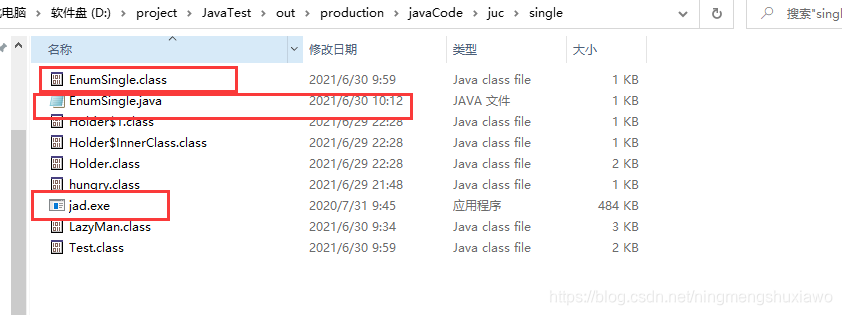
// Decompiled by Jad v1.5.8g. Copyright 2001 Pavel Kouznetsov.
// Jad home page: http://www.kpdus.com/jad.html
// Decompiler options: packimports(3)
// Source File Name: EnumSingle.java
package juc.single;
public final class EnumSingle extends Enum
{
public static EnumSingle[] values()
{
return (EnumSingle[])$VALUES.clone();
}
public static EnumSingle valueOf(String name)
{
return (EnumSingle)Enum.valueOf(juc/single/EnumSingle, name);
}
private EnumSingle(String s, int i)
{
super(s, i);
}
public static EnumSingle getInstance()
{
return INSTANCE;
}
public static final EnumSingle INSTANCE;
private static final EnumSingle $VALUES[];
static
{
INSTANCE = new EnumSingle("INSTANCE", 0);
$VALUES = (new EnumSingle[] {
INSTANCE
});
}
}
可以看到,不是无参构造器哦,而是有参构造器,有一个String,一个Int
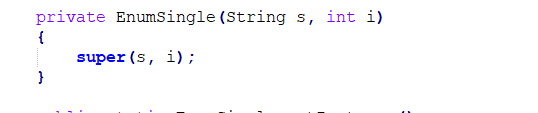
现在我们修改反射代码
Constructor<EnumSingle> declaredConstructor = EnumSingle.class.getDeclaredConstructor(String.class,int.class);
得到我们想要的结果了,抛出反射不能破坏枚举的单例异常

public enum EnumSingle {
INSTANCE;
public static EnumSingle getInstance()
{
return INSTANCE;
}
}
class Test{
public static void main(String[] args) throws NoSuchMethodException, IllegalAccessException, InvocationTargetException, InstantiationException {
EnumSingle instance1=EnumSingle.INSTANCE;
// EnumSingle instance2=EnumSingle.INSTANCE;
// EnumSingle instance3=EnumSingle.getInstance();
// Constructor<EnumSingle> declaredConstructor = EnumSingle.class.getDeclaredConstructor(null);
Constructor<EnumSingle> declaredConstructor = EnumSingle.class.getDeclaredConstructor(String.class,int.class);
declaredConstructor.setAccessible(true);//先把构造器的私有权限破除,使得反射可以访问,创建对象
EnumSingle instance2= declaredConstructor.newInstance();
System.out.println( instance1);
System.out.println( instance2);
}
}
15. 理解CAS
1)什么是CAS?
CAS 是一种硬件级别的原子操作,它比较内存中的某个值是否为预期值,如果是,则更新为新值,否则不做修改。
工作原理:
- 比较(Compare):CAS 会检查内存中的某个值是否与预期值相等。
- 交换(Swap):如果相等,则将内存中的值更新为新值。
- 失败重试:如果不相等,说明有其他线程已经修改了该值,CAS 操作失败,一般会利用重试,直到成功。
举例说明
我们经常有累加需求,比较一个值是否等于 1,如果等于 1 我们将它替换成 2,如果等于 2 替换成 3。
这种比较在多线程的情况下就不安全,比如此时同时有两个线程执行到比较值是否等于 1,然后两个线程发现都等于 1。
然后两个线程都将它变成了 2,这样明明加了两次,值却等于 2。
这种情况其实加锁可以解决,但是加锁是比较消耗资源的。
因此硬件层面就给予支持,将这个比较和交换的动作封装成一个指令,这样就保证了原子性,不会判断值确实等于 1,但是替换的时候值已经不等于 1了。
这指令就是 CAS。
public class casDemo {
//CAS : compareAndSet 比较并交换
public static void main(String[] args) {
AtomicInteger atomicInteger = new AtomicInteger(2020);
//boolean compareAndSet(int expect, int update)
//期望值、更新值
//如果实际值 和 我的期望值相同,那么就更新
//如果实际值 和 我的期望值不同,那么就不更新
System.out.println(atomicInteger.compareAndSet(2020, 2021));
System.out.println(atomicInteger.get());
//因为期望值是2020 实际值却变成了2021 所以会修改失败
//CAS 是CPU的并发原语
atomicInteger.getAndIncrement(); //++操作
System.out.println(atomicInteger.compareAndSet(2020, 2021));
System.out.println(atomicInteger.get());
}
}
Java无法操作内存,但是C++可以操作内存,Java可以通过native方法调用c++从而操作内存

Unsafe 类
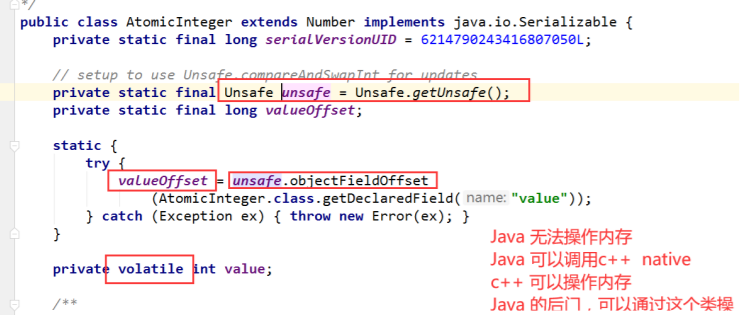
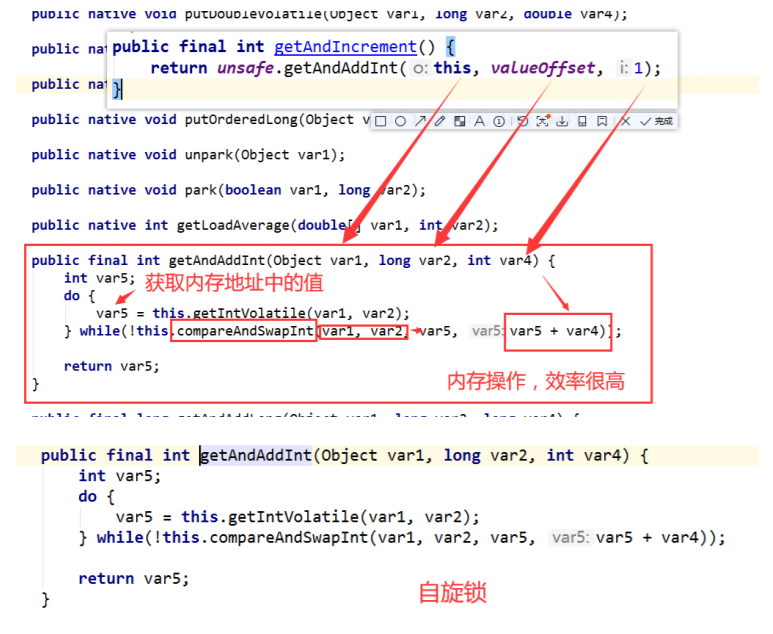
总结:CAS:比较当前工作内存中的值和主内存中的值,如果这个值是期望的,那么则执行操作,如果不是,就一直循环,使用的是自旋锁。因为比较并交换的过程是必须是原子性的,所以底层是通过在cpu指令上完成cas操作的;
好处:是不用切换线程状态,因为切换线程状态性能消耗比较大
缺点:
1:由于底层是自旋锁,循环会浪费时间
2:因为是底层的cpu操作,一次只能保证一个共享变量的原子性
3:ABA问题
16.原子引用解决ABA问题
CAS:ABA问题?(狸猫换太子)
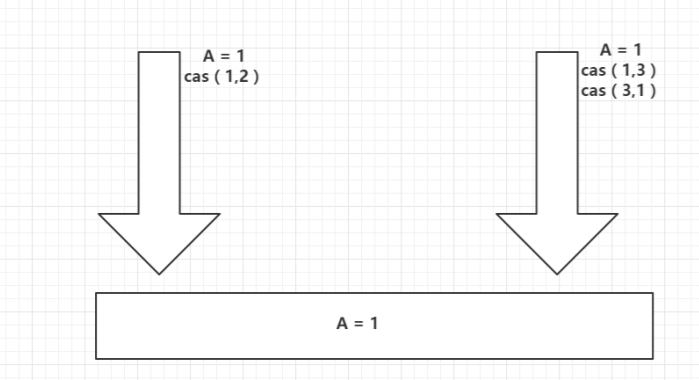
线程1:期望值是1,要变成2;
线程2:两个操作:
- 1、期望值是1,变成3
- 2、期望是3,变成1
所以对于线程1来说,A的值还是1,所以就出现了问题,骗过了线程1;
public class casDemo {
//CAS : compareAndSet 比较并交换
public static void main(String[] args) {
AtomicInteger atomicInteger = new AtomicInteger(2020);
System.out.println(atomicInteger.compareAndSet(2020, 2021));
System.out.println(atomicInteger.get());
//boolean compareAndSet(int expect, int update)
//期望值、更新值
//如果实际值 和 我的期望值相同,那么就更新
//如果实际值 和 我的期望值不同,那么就不更新
System.out.println(atomicInteger.compareAndSet(2021, 2020));
System.out.println(atomicInteger.get());
//因为期望值是2020 实际值却变成了2021 所以会修改失败
//CAS 是CPU的并发原语
// atomicInteger.getAndIncrement(); //++操作
System.out.println(atomicInteger.compareAndSet(2020, 2021));
System.out.println(atomicInteger.get());
}
}
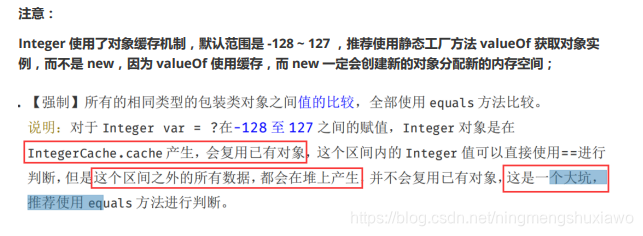
public class CASDemo {
/**AtomicStampedReference 注意,如果泛型是一个包装类,注意对象的引用问题
* 正常在业务操作,这里面比较的都是一个个对象
*/
static AtomicStampedReference<Integer> atomicStampedReference = new
AtomicStampedReference<>(1, 1);
// CAS compareAndSet : 比较并交换!
public static void main(String[] args) {
new Thread(() -> {
int stamp = atomicStampedReference.getStamp(); // 获得版本号
System.out.println("a1=>" + stamp);
try {
TimeUnit.SECONDS.sleep(1);
} catch (InterruptedException e) {
e.printStackTrace();
}
// 修改操作时,版本号更新 + 1
atomicStampedReference.compareAndSet(1, 2,
atomicStampedReference.getStamp(),
atomicStampedReference.getStamp() + 1);
System.out.println("a2=>" + atomicStampedReference.getStamp());
// 重新把值改回去, 版本号更新 + 1
System.out.println(atomicStampedReference.compareAndSet(2, 1,
atomicStampedReference.getStamp(),
atomicStampedReference.getStamp() + 1));
System.out.println("a3=>" + atomicStampedReference.getStamp());
}, "a").start();
// 乐观锁的原理相同!
new Thread(() -> {
int stamp = atomicStampedReference.getStamp(); // 获得版本号
System.out.println("b1=>" + stamp);
try {
TimeUnit.SECONDS.sleep(2);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println(atomicStampedReference.compareAndSet(1, 3,
stamp, stamp + 1));
System.out.println("b2=>" + atomicStampedReference.getStamp());
}, "b").start();
}
}
17. 各种锁的理解
1)公平锁,非公平锁
- 公平锁:非常公平,不能插队,必须先来后到
/**
* Creates an instance of {@code ReentrantLock}.
* This is equivalent to using {@code ReentrantLock(false)}.
*/
public ReentrantLock() {
sync = new NonfairSync();
}
- 非公平锁:非常不公平,允许插队,可以改变顺序,synchronized和lock默认都是非公平锁
/**
* Creates an instance of {@code ReentrantLock} with the
* given fairness policy.
*
* @param fair {@code true} if this lock should use a fair ordering policy
*/
public ReentrantLock(boolean fair) {
sync = fair ? new FairSync() : new NonfairSync();
}
2)可重入锁
所有的锁都是可重入锁,有些地方叫做递归锁
你进入你家,拿到了大门的锁,也就自动拿到了里面小门的锁
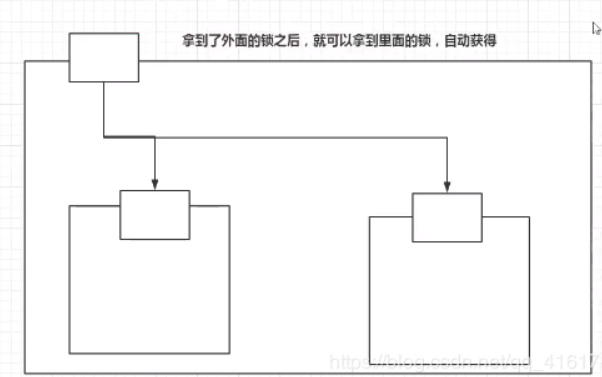
- Synchonized 锁
public class Demo01 {
public static void main(String[] args) {
Phone phone = new Phone();
new Thread(()->{
phone.sms();
},"A").start();
new Thread(()->{
phone.sms();
},"B").start();
}
}
class Phone{
public synchronized void sms(){
System.out.println(Thread.currentThread().getName()+"=> sms");
call();//这里也有一把锁
}
public synchronized void call(){
System.out.println(Thread.currentThread().getName()+"=> call");
}
}
- Lock 锁
//lock
public class Demo02 {
public static void main(String[] args) {
Phone2 phone = new Phone2();
new Thread(()->{
phone.sms();
},"A").start();
new Thread(()->{
phone.sms();
},"B").start();
}
}
class Phone2{
Lock lock=new ReentrantLock();
public void sms(){
lock.lock(); //细节:这个是两把锁,两个钥匙
//lock锁必须配对,否则就会死锁在里面
try {
System.out.println(Thread.currentThread().getName()+"=> sms");
call();//这里也有一把锁
} catch (Exception e) {
e.printStackTrace();
}finally {
lock.unlock();
}
}
public void call(){
lock.lock();
try {
System.out.println(Thread.currentThread().getName() + "=> call");
}catch (Exception e){
e.printStackTrace();
}
finally {
lock.unlock();
}
}
}
- lock锁必须配对,相当于lock和 unlock 必须数量相同;
- 在外面加的锁,也可以在里面解锁;在里面加的锁,在外面也可以解锁;
3)自旋锁
- spinlock
public final int getAndAddInt(Object var1, long var2, int var4) {
int var5;
do {
var5 = this.getIntVolatile(var1, var2);
} while(!this.compareAndSwapInt(var1, var2, var5, var5 + var4));
return var5;
}
- 自我设计自旋锁
public class SpinlockDemo {
// 默认
// int 0
//thread null
AtomicReference<Thread> atomicReference=new AtomicReference<>();
//加锁
public void myLock(){
Thread thread = Thread.currentThread();
System.out.println(thread.currentThread().getName()+"===> mylock");
//自旋锁
while (!atomicReference.compareAndSet(null,thread)){
System.out.println(Thread.currentThread().getName()+" ==> 自旋中~");
}
}
//解锁
public void myUnlock(){
Thread thread=Thread.currentThread();
System.out.println(thread.currentThread().getName()+"===> myUnlock");
atomicReference.compareAndSet(thread,null);
}
}
public class TestSpinLock {
public static void main(String[] args) throws InterruptedException {
//ReentrantLock reentrantLock = new ReentrantLock();
//reentrantLock.lock();
//reentrantLock.unlock();
//使用CAS实现自旋锁
SpinlockDemo spinlockDemo=new SpinlockDemo();
new Thread(()->{
spinlockDemo.myLock();
try {
TimeUnit.SECONDS.sleep(3);
} catch (Exception e) {
e.printStackTrace();
} finally {
spinlockDemo.myUnlock();
}
},"t1").start();
TimeUnit.SECONDS.sleep(1);
new Thread(()->{
spinlockDemo.myLock();
try {
TimeUnit.SECONDS.sleep(3);
} catch (Exception e) {
e.printStackTrace();
} finally {
spinlockDemo.myUnlock();
}
},"t2").start();
}
}
t2进程必须等待t1进程Unlock后,才能Unlock,在这之前进行自旋等待
4)死锁
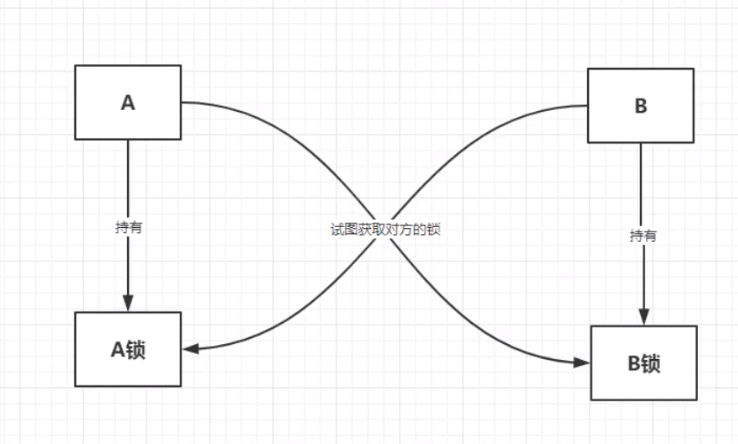
public class DeadLock {
public static void main(String[] args) {
String lockA= "lockA";
String lockB= "lockB";
new Thread(new MyThread(lockA,lockB),"t1").start();
new Thread(new MyThread(lockB,lockA),"t2").start();
}
}
class MyThread implements Runnable{
private String lockA;
private String lockB;
public MyThread(String lockA, String lockB) {
this.lockA = lockA;
this.lockB = lockB;
}
@Override
public void run() {
synchronized (lockA){
System.out.println(Thread.currentThread().getName()+" lock"+lockA+"===>get"+lockB);
try {
TimeUnit.SECONDS.sleep(2);
} catch (InterruptedException e) {
e.printStackTrace();
}
synchronized (lockB){
System.out.println(Thread.currentThread().getName()+" lock"+lockB+"===>get"+lockA);
}
}
}
}
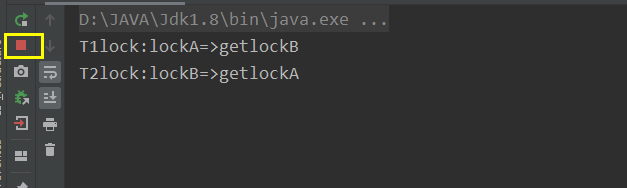
jps定位进程号,查看哪一个进程出了问题
g var2, int var4) {
int var5;
do {
var5 = this.getIntVolatile(var1, var2);
} while(!this.compareAndSwapInt(var1, var2, var5, var5 + var4));
return var5;
}
2. 自我设计自旋锁
```java
public class SpinlockDemo {
// 默认
// int 0
//thread null
AtomicReference<Thread> atomicReference=new AtomicReference<>();
//加锁
public void myLock(){
Thread thread = Thread.currentThread();
System.out.println(thread.currentThread().getName()+"===> mylock");
//自旋锁
while (!atomicReference.compareAndSet(null,thread)){
System.out.println(Thread.currentThread().getName()+" ==> 自旋中~");
}
}
//解锁
public void myUnlock(){
Thread thread=Thread.currentThread();
System.out.println(thread.currentThread().getName()+"===> myUnlock");
atomicReference.compareAndSet(thread,null);
}
}
public class TestSpinLock {
public static void main(String[] args) throws InterruptedException {
//ReentrantLock reentrantLock = new ReentrantLock();
//reentrantLock.lock();
//reentrantLock.unlock();
//使用CAS实现自旋锁
SpinlockDemo spinlockDemo=new SpinlockDemo();
new Thread(()->{
spinlockDemo.myLock();
try {
TimeUnit.SECONDS.sleep(3);
} catch (Exception e) {
e.printStackTrace();
} finally {
spinlockDemo.myUnlock();
}
},"t1").start();
TimeUnit.SECONDS.sleep(1);
new Thread(()->{
spinlockDemo.myLock();
try {
TimeUnit.SECONDS.sleep(3);
} catch (Exception e) {
e.printStackTrace();
} finally {
spinlockDemo.myUnlock();
}
},"t2").start();
}
}
t2进程必须等待t1进程Unlock后,才能Unlock,在这之前进行自旋等待
4)死锁
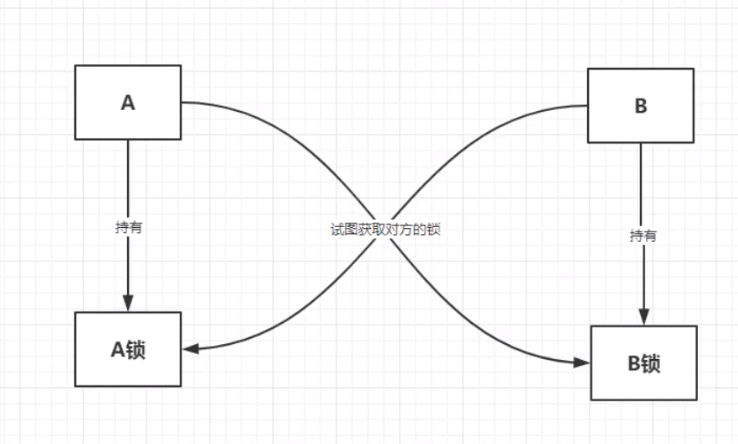
public class DeadLock {
public static void main(String[] args) {
String lockA= "lockA";
String lockB= "lockB";
new Thread(new MyThread(lockA,lockB),"t1").start();
new Thread(new MyThread(lockB,lockA),"t2").start();
}
}
class MyThread implements Runnable{
private String lockA;
private String lockB;
public MyThread(String lockA, String lockB) {
this.lockA = lockA;
this.lockB = lockB;
}
@Override
public void run() {
synchronized (lockA){
System.out.println(Thread.currentThread().getName()+" lock"+lockA+"===>get"+lockB);
try {
TimeUnit.SECONDS.sleep(2);
} catch (InterruptedException e) {
e.printStackTrace();
}
synchronized (lockB){
System.out.println(Thread.currentThread().getName()+" lock"+lockB+"===>get"+lockA);
}
}
}
}
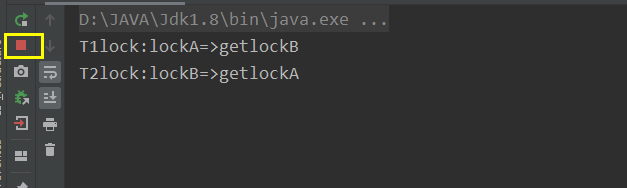
jps定位进程号,查看哪一个进程出了问题
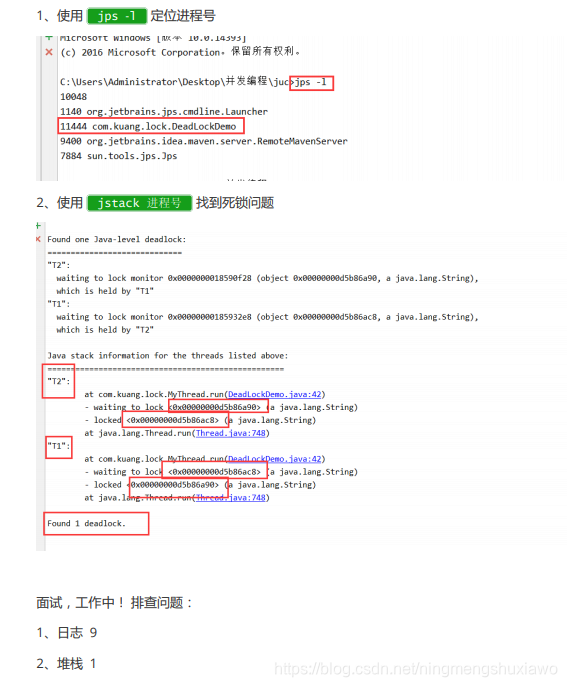